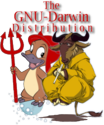
|
Image class for encapsulating the storage texture image data
Inheritance:
Public Methods-
Image()
-
Image(const Image& image, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp& copyop) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
void setFileName(const std::string& fileName)
-
inline const std::string& getFileName() const
-
void allocateImage(int s, int t, int r, GLenum format, GLenum type, int packing=1)
-
void setImage(int s, int t, int r, GLint internalTextureformat, GLenum format, GLenum type, unsigned char* data, int packing=1)
- set the image data and format.
-
void readPixels(int x, int y, int width, int height, GLenum format, GLenum type)
- readPixels from screen at specified position and size, using glReadPixels.
-
void scaleImage(int s, int t, int r)
- Scale image to specified size.
-
void copySubImage(int s_offset, int t_offset, int r_offset, osg::Image* source)
- Copy a source Image into a subpart of this Image at specified position.
-
inline int s() const
- Width of image
-
inline int t() const
- Height of image
-
inline int r() const
- Depth of image
-
void setInternalTextureFormat(GLint internalFormat)
-
inline GLint getInternalTextureFormat() const
-
void setPixelFormat(GLenum format)
-
inline GLenum getPixelFormat() const
-
inline GLenum getDataType() const
-
inline unsigned int getPacking() const
-
inline unsigned int getPixelSizeInBits() const
- return the numbers of bits required for each pixel
-
inline unsigned int getRowSizeInBytes() const
- return the numbers of bytes each row of pixels occupies once it has been packed
-
inline unsigned int getImageSizeInBytes() const
- return the numbers of bytes each image (_s*_t) of pixels occupies
-
inline unsigned int getTotalSizeInBytes() const
- return the numbers of bytes the whole row/image/volume of pixels occupies
-
inline unsigned char* data()
- raw image data
-
inline const unsigned char* data() const
- raw const image data
-
inline unsigned char* data(int column, int row=0, int image=0) const
-
void flipHorizontal(int image=0)
- Flip the image horizontally
-
void flipVertical(int image=0)
- Flip the image vertically
-
void ensureValidSizeForTexturing(GLint maxTextureSize)
- Ensure image dimensions are a power of two.
-
inline void dirty()
- Dirty the image, which increments the modified flag, to force osg::Texture to reload the image
-
inline void setModifiedTag(unsigned int value)
- Set the modified tag value, only used by osg::Texture when using texture subloading.
-
inline unsigned int getModifiedTag() const
- Get modified tag value, only used by osg::Texture when using texture subloading.
-
static bool isPackedType(GLenum type)
-
static unsigned int computeNumComponents(GLenum format)
-
static unsigned int computePixelSizeInBits(GLenum format, GLenum type)
-
static unsigned int computeRowWidthInBytes(int width, GLenum format, GLenum type, int packing)
-
static unsigned int computeNearestPowerOfTwo(unsigned int s, float bias=0.5f)
-
inline bool isMipmap() const
-
unsigned int getNumMipmapLevels() const
-
inline void setMipmapData(const MipmapDataType& mipmapDataVector)
-
inline unsigned char* getMipmapData(unsigned int mipmapNumber) const
-
void computeMipMaps()
- converts a single image into mip mapped version image
-
bool isImageTranslucent() const
- return true of this image is translucent - ie.
Public Members-
typedef std::vector< unsigned int > MipmapDataType
Protected Fields-
std::string _fileName
-
int _s
-
int _t
-
int _r
-
GLint _internalTextureFormat
-
GLenum _pixelFormat
-
GLenum _dataType
-
unsigned int _packing
-
unsigned char* _data
-
unsigned int _modifiedTag
-
MipmapDataType _mipmapData
Protected Methods-
virtual ~Image()
-
Image& operator = (const Image&)
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Image class for encapsulating the storage texture image data
Image()
Image(const Image& image, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
virtual Object* cloneType() const
virtual Object* clone(const CopyOp& copyop) const
virtual bool isSameKindAs(const Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
void setFileName(const std::string& fileName)
inline const std::string& getFileName() const
void allocateImage(int s, int t, int r, GLenum format, GLenum type, int packing=1)
void setImage(int s, int t, int r, GLint internalTextureformat, GLenum format, GLenum type, unsigned char* data, int packing=1)
- set the image data and format.
note, when the packing value is negative (the default is -1) this method assumes
a _packing width of 1 if the width is not a multiple of 4,
otherwise automatically sets to _packing to 4. If a positive
value of packing is supplied than _packing is simply set to that value.
void readPixels(int x, int y, int width, int height, GLenum format, GLenum type)
- readPixels from screen at specified position and size, using glReadPixels.
Create memory for storage if required, reuse existing pixel coords if possible.
if pixelFormat or dataType
void scaleImage(int s, int t, int r)
- Scale image to specified size.
void copySubImage(int s_offset, int t_offset, int r_offset, osg::Image* source)
- Copy a source Image into a subpart of this Image at specified position.
Typically used to copy to an already allocated image, such as creating
a 3D image from a stack 2D images.
If the this Image is empty then image data is created to
accomodate the imaging image in its offset position.
If source is NULL then no operation happens, this Image is left unchanged.
inline int s() const
- Width of image
inline int t() const
- Height of image
inline int r() const
- Depth of image
void setInternalTextureFormat(GLint internalFormat)
inline GLint getInternalTextureFormat() const
void setPixelFormat(GLenum format)
inline GLenum getPixelFormat() const
inline GLenum getDataType() const
inline unsigned int getPacking() const
inline unsigned int getPixelSizeInBits() const
- return the numbers of bits required for each pixel
inline unsigned int getRowSizeInBytes() const
- return the numbers of bytes each row of pixels occupies once it has been packed
inline unsigned int getImageSizeInBytes() const
- return the numbers of bytes each image (_s*_t) of pixels occupies
inline unsigned int getTotalSizeInBytes() const
- return the numbers of bytes the whole row/image/volume of pixels occupies
inline unsigned char* data()
- raw image data
inline const unsigned char* data() const
- raw const image data
inline unsigned char* data(int column, int row=0, int image=0) const
void flipHorizontal(int image=0)
- Flip the image horizontally
void flipVertical(int image=0)
- Flip the image vertically
void ensureValidSizeForTexturing(GLint maxTextureSize)
- Ensure image dimensions are a power of two.
Mip Mapped texture require the image dimensions to be
power of two and are within the maxiumum texture size for
the host machine.
inline void dirty()
- Dirty the image, which increments the modified flag, to force osg::Texture to reload the image
inline void setModifiedTag(unsigned int value)
- Set the modified tag value, only used by osg::Texture when using texture subloading.
inline unsigned int getModifiedTag() const
- Get modified tag value, only used by osg::Texture when using texture subloading.
static bool isPackedType(GLenum type)
static unsigned int computeNumComponents(GLenum format)
static unsigned int computePixelSizeInBits(GLenum format, GLenum type)
static unsigned int computeRowWidthInBytes(int width, GLenum format, GLenum type, int packing)
static unsigned int computeNearestPowerOfTwo(unsigned int s, float bias=0.5f)
typedef std::vector< unsigned int > MipmapDataType
inline bool isMipmap() const
unsigned int getNumMipmapLevels() const
inline void setMipmapData(const MipmapDataType& mipmapDataVector)
inline unsigned char* getMipmapData(unsigned int mipmapNumber) const
void computeMipMaps()
- converts a single image into mip mapped version image
bool isImageTranslucent() const
- return true of this image is translucent - ie. it has alpha values that are less 1.0 (when normalized).
virtual ~Image()
Image& operator = (const Image&)
std::string _fileName
int _s
int _t
int _r
GLint _internalTextureFormat
GLenum _pixelFormat
GLenum _dataType
unsigned int _packing
unsigned char* _data
unsigned int _modifiedTag
MipmapDataType _mipmapData
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|