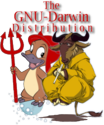
|
A plane class.
Inheritance:
Public Methods-
inline Plane()
-
inline Plane(const Plane& pl)
-
inline Plane(float a, float b, float c, float d)
-
inline Plane(const Vec4& vec)
-
inline Plane(const Vec3& norm, float d)
-
inline Plane(const Vec3& v1, const Vec3& v2, const Vec3& v3)
-
inline Plane& operator = (const Plane& pl)
-
inline void set(const Plane& pl)
-
inline void set(float a, float b, float c, float d)
-
inline void set(const Vec4& vec)
-
inline void set(const Vec3& norm, float d)
-
inline void set(const Vec3& v1, const Vec3& v2, const Vec3& v3)
-
inline void set(const Vec3& norm, const Vec3& point)
-
inline void flip()
- flip/reverse the orientation of the plane
-
inline void makeUnitLength()
-
inline void calculateUpperLowerBBCorners()
- calculate the upper and lower bounding box corners to be used in the intersect(BoundingBox&) method for speeding calculations
-
inline bool valid() const
-
inline bool operator == (const Plane& plane) const
-
inline bool operator != (const Plane& plane) const
-
inline bool operator < (const Plane& plane) const
-
inline float* ptr()
-
inline const float* ptr() const
-
inline Vec4& asVec4()
-
inline const Vec4& asVec4() const
-
inline float& operator [] (unsigned int i)
-
inline float operator [] (unsigned int i) const
-
inline osg::Vec3 getNormal() const
-
inline float distance(const osg::Vec3& v) const
- calculate the distance between a point and the plane
-
inline int intersect(const std::vector<Vec3>& vertices) const
- intersection test between plane and vertex list return 1 if the bs is completely above plane, return 0 if the bs intersects the plane, return -1 if the bs is completely below the plane
-
inline int intersect(const BoundingSphere& bs) const
- intersection test between plane and bounding sphere.
-
inline int intersect(const BoundingBox& bb) const
- intersection test between plane and bounding sphere.
-
inline void transform(const osg::Matrix& matrix)
- Transform the plane by matrix.
-
inline void transformProvidingInverse(const osg::Matrix& matrix)
- Transform the plane by provide a pre inverted matrix.
Protected Fields-
Vec4 _fv
-
unsigned int _upperBBCorner
-
unsigned int _lowerBBCorner
Documentation
A plane class. It can be used to represent an infinite plane.
inline Plane()
inline Plane(const Plane& pl)
inline Plane(float a, float b, float c, float d)
inline Plane(const Vec4& vec)
inline Plane(const Vec3& norm, float d)
inline Plane(const Vec3& v1, const Vec3& v2, const Vec3& v3)
inline Plane& operator = (const Plane& pl)
inline void set(const Plane& pl)
inline void set(float a, float b, float c, float d)
inline void set(const Vec4& vec)
inline void set(const Vec3& norm, float d)
inline void set(const Vec3& v1, const Vec3& v2, const Vec3& v3)
inline void set(const Vec3& norm, const Vec3& point)
inline void flip()
- flip/reverse the orientation of the plane
inline void makeUnitLength()
inline void calculateUpperLowerBBCorners()
- calculate the upper and lower bounding box corners to be used
in the intersect(BoundingBox&) method for speeding calculations
inline bool valid() const
inline bool operator == (const Plane& plane) const
inline bool operator != (const Plane& plane) const
inline bool operator < (const Plane& plane) const
inline float* ptr()
inline const float* ptr() const
inline Vec4& asVec4()
inline const Vec4& asVec4() const
inline float& operator [] (unsigned int i)
inline float operator [] (unsigned int i) const
inline osg::Vec3 getNormal() const
inline float distance(const osg::Vec3& v) const
- calculate the distance between a point and the plane
inline int intersect(const std::vector<Vec3>& vertices) const
- intersection test between plane and vertex list
return 1 if the bs is completely above plane,
return 0 if the bs intersects the plane,
return -1 if the bs is completely below the plane
inline int intersect(const BoundingSphere& bs) const
- intersection test between plane and bounding sphere.
return 1 if the bs is completely above plane,
return 0 if the bs intersects the plane,
return -1 if the bs is completely below the plane.
inline int intersect(const BoundingBox& bb) const
- intersection test between plane and bounding sphere.
return 1 if the bs is completely above plane,
return 0 if the bs intersects the plane,
return -1 if the bs is completely below the plane.
inline void transform(const osg::Matrix& matrix)
- Transform the plane by matrix. Note, this operations carries out
the calculation of the inverse of the matrix since to transforms
planes must be multiplied my the inverse transposed. This
make this operation expensive. If the inverse has been already
calculated elsewhere then use transformProvidingInverse() instead.
See http://www.worldserver.com/turk/computergraphics/NormalTransformations.pdf
inline void transformProvidingInverse(const osg::Matrix& matrix)
- Transform the plane by provide a pre inverted matrix.
see transform for details.
Vec4 _fv
unsigned int _upperBBCorner
unsigned int _lowerBBCorner
- Direct child classes:
- InfinitePlane
- Friends:
- inline std::ostream& operator << (std::ostream& output, const Plane& pl)
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|