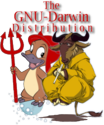
|
Inheritance:
Public Methods-
Matrix()
-
Matrix( const Matrix& other)
-
explicit Matrix( float const* const def )
-
Matrix( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
-
~Matrix()
-
int compare(const Matrix& m) const
-
bool operator < (const Matrix& m) const
-
bool operator == (const Matrix& m) const
-
bool operator != (const Matrix& m) const
-
inline float& operator()(int row, int col)
-
inline float operator()(int row, int col) const
-
inline bool valid() const
-
inline bool isNaN() const
-
inline Matrix& operator = (const Matrix& other)
-
inline void set(const Matrix& other)
-
inline void set(float const* const ptr)
-
void set( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
-
float* ptr() const
-
void makeIdentity()
-
void makeScale( const Vec3& )
-
void makeScale( float, float, float )
-
void makeTranslate( const Vec3& )
-
void makeTranslate( float, float, float )
-
void makeRotate( const Vec3& from, const Vec3& to )
-
void makeRotate( float angle, const Vec3& axis )
-
void makeRotate( float angle, float x, float y, float z )
-
void makeRotate( const Quat& )
-
void makeRotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
void makeOrtho(double left, double right, double bottom, double top, double zNear, double zFar)
- Set to a orthographic projection.
-
inline void makeOrtho2D(double left, double right, double bottom, double top)
- Set to a 2D orthographic projection.
-
void makeFrustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Set to a perspective projection.
-
void makePerspective(double fovy, double aspectRatio, double zNear, double zFar)
- Set to a symmetrical perspective projection, See gluPerspective for further details.
-
void makeLookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- Set to the position and orientation as per a camera, using the same convention as gluLookAt.
-
bool invert( const Matrix& )
-
inline static Matrix identity( void )
-
inline static Matrix scale( const Vec3& sv)
-
inline static Matrix scale( float sx, float sy, float sz)
-
inline static Matrix translate( const Vec3& dv)
-
inline static Matrix translate( float x, float y, float z)
-
inline static Matrix rotate( const Vec3& from, const Vec3& to)
-
inline static Matrix rotate( float angle, float x, float y, float z)
-
inline static Matrix rotate( float angle, const Vec3& axis)
-
inline static Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
inline static Matrix rotate( const Quat& quat)
-
inline static Matrix inverse( const Matrix& matrix)
-
inline static Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
- Create a orthographic projection.
-
inline static Matrix ortho2D(double left, double right, double bottom, double top)
- Create a 2D orthographic projection.
-
inline static Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Create a perspective projection.
-
inline static Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
- Create a symmetrical perspective projection, See gluPerspective for further details.
-
inline static Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- Create the position and orientation as per a camera, using the same convention as gluLookAt.
-
void setTrans( float tx, float ty, float tz )
-
void setTrans( const Vec3& v )
-
inline Vec3 getTrans() const
-
inline Vec3 getScale() const
-
inline static Vec3 transform3x3(const Vec3& v, const Matrix& m)
- apply apply an 3x3 transform of v*M[02,02]
-
inline static Vec3 transform3x3(const Matrix& m, const Vec3& v)
- apply apply an 3x3 transform of M[02,02]*v
-
void mult( const Matrix&, const Matrix& )
-
void preMult( const Matrix& )
-
void postMult( const Matrix& )
-
inline void operator *= ( const Matrix& other )
-
inline Matrix operator * ( const Matrix &m ) const
-
inline Matrix identity(void)
-
inline Matrix scale(float sx, float sy, float sz)
-
inline Matrix scale(const Vec3& v )
-
inline Matrix translate(float tx, float ty, float tz)
-
inline Matrix translate(const Vec3& v )
-
inline Matrix rotate( const Quat& q )
-
inline Matrix rotate(float angle, float x, float y, float z )
-
inline Matrix rotate(float angle, const Vec3& axis )
-
inline Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
inline Matrix rotate(const Vec3& from, const Vec3& to )
-
inline Matrix inverse( const Matrix& matrix)
-
inline Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline Matrix ortho2D(double left, double right, double bottom, double top)
-
inline Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
-
inline Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
-
inline Vec3 postMult( const Vec3& v ) const
-
inline Vec3 preMult( const Vec3& v ) const
-
inline Vec4 postMult( const Vec4& v ) const
-
inline Vec4 preMult( const Vec4& v ) const
-
inline Vec3 transform3x3(const Vec3& v, const Matrix& m)
-
inline Vec3 transform3x3(const Matrix& m, const Vec3& v)
-
inline Vec3 operator* (const Vec3& v) const
-
inline Vec4 operator* (const Vec4& v) const
Protected Fields-
float _mat[4][4]
Documentation
Matrix()
Matrix( const Matrix& other)
explicit Matrix( float const* const def )
Matrix( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
~Matrix()
int compare(const Matrix& m) const
bool operator < (const Matrix& m) const
bool operator == (const Matrix& m) const
bool operator != (const Matrix& m) const
inline float& operator()(int row, int col)
inline float operator()(int row, int col) const
inline bool valid() const
inline bool isNaN() const
inline Matrix& operator = (const Matrix& other)
inline void set(const Matrix& other)
inline void set(float const* const ptr)
void set( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
float* ptr() const
void makeIdentity()
void makeScale( const Vec3& )
void makeScale( float, float, float )
void makeTranslate( const Vec3& )
void makeTranslate( float, float, float )
void makeRotate( const Vec3& from, const Vec3& to )
void makeRotate( float angle, const Vec3& axis )
void makeRotate( float angle, float x, float y, float z )
void makeRotate( const Quat& )
void makeRotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
void makeOrtho(double left, double right, double bottom, double top, double zNear, double zFar)
- Set to a orthographic projection. See glOrtho for further details.
inline void makeOrtho2D(double left, double right, double bottom, double top)
- Set to a 2D orthographic projection. See glOrtho2D for further details.
void makeFrustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Set to a perspective projection. See glFrustum for further details.
void makePerspective(double fovy, double aspectRatio, double zNear, double zFar)
- Set to a symmetrical perspective projection, See gluPerspective for further details.
Aspect ratio is defined as width/height.
void makeLookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- Set to the position and orientation as per a camera, using the same convention as gluLookAt.
bool invert( const Matrix& )
inline static Matrix identity( void )
inline static Matrix scale( const Vec3& sv)
inline static Matrix scale( float sx, float sy, float sz)
inline static Matrix translate( const Vec3& dv)
inline static Matrix translate( float x, float y, float z)
inline static Matrix rotate( const Vec3& from, const Vec3& to)
inline static Matrix rotate( float angle, float x, float y, float z)
inline static Matrix rotate( float angle, const Vec3& axis)
inline static Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
inline static Matrix rotate( const Quat& quat)
inline static Matrix inverse( const Matrix& matrix)
inline static Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
- Create a orthographic projection. See glOrtho for further details.
inline static Matrix ortho2D(double left, double right, double bottom, double top)
- Create a 2D orthographic projection. See glOrtho for further details.
inline static Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Create a perspective projection. See glFrustum for further details.
inline static Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
- Create a symmetrical perspective projection, See gluPerspective for further details.
Aspect ratio is defined as width/height.
inline static Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- Create the position and orientation as per a camera, using the same convention as gluLookAt.
void setTrans( float tx, float ty, float tz )
void setTrans( const Vec3& v )
inline Vec3 getTrans() const
inline Vec3 getScale() const
inline static Vec3 transform3x3(const Vec3& v, const Matrix& m)
- apply apply an 3x3 transform of v*M[02,02]
inline static Vec3 transform3x3(const Matrix& m, const Vec3& v)
- apply apply an 3x3 transform of M[02,02]*v
void mult( const Matrix&, const Matrix& )
void preMult( const Matrix& )
void postMult( const Matrix& )
inline void operator *= ( const Matrix& other )
inline Matrix operator * ( const Matrix &m ) const
float _mat[4][4]
inline Matrix identity(void)
inline Matrix scale(float sx, float sy, float sz)
inline Matrix scale(const Vec3& v )
inline Matrix translate(float tx, float ty, float tz)
inline Matrix translate(const Vec3& v )
inline Matrix rotate( const Quat& q )
inline Matrix rotate(float angle, float x, float y, float z )
inline Matrix rotate(float angle, const Vec3& axis )
inline Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
inline Matrix rotate(const Vec3& from, const Vec3& to )
inline Matrix inverse( const Matrix& matrix)
inline Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
inline Matrix ortho2D(double left, double right, double bottom, double top)
inline Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
inline Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
inline Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
inline Vec3 postMult( const Vec3& v ) const
inline Vec3 preMult( const Vec3& v ) const
inline Vec4 postMult( const Vec4& v ) const
inline Vec4 preMult( const Vec4& v ) const
inline Vec3 transform3x3(const Vec3& v, const Matrix& m)
inline Vec3 transform3x3(const Matrix& m, const Vec3& v)
inline Vec3 operator* (const Vec3& v) const
inline Vec4 operator* (const Vec4& v) const
- Direct child classes:
- RefMatrix
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|