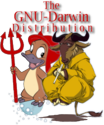
|
Light state class which encapsulates OpenGL glLight() functionality
Inheritance:
Public Methods-
Light()
-
Light(const Light& light, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
META_StateAttribute(osg, Light, (Type)(LIGHT_0+_lightnum))
-
virtual int compare(const StateAttribute& sa) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
-
virtual void getAssociatedModes(std::vector<GLMode>& modes) const
-
void setLightNum(int num)
- Set which OpenGL light to operate on
-
int getLightNum() const
- Get which OpenGL light this osg::Light operates on
-
inline void setAmbient( const Vec4& ambient )
- Set the ambient component of the light.
-
inline const Vec4& getAmbient() const
- Get the ambient component of the light.
-
inline void setDiffuse( const Vec4& diffuse )
- Set the diffuse component of the light.
-
inline const Vec4& getDiffuse() const
- Get the diffuse component of the light.
-
inline void setSpecular( const Vec4& specular )
- Set the specular component of the light.
-
inline const Vec4& getSpecular() const
- Get the specular component of the light.
-
inline void setPosition( const Vec4& position )
- Set the position of the light.
-
inline const Vec4& getPosition() const
- Get the position of the light.
-
inline void setDirection( const Vec3& direction )
- Set the direction of the light.
-
inline const Vec3& getDirection() const
- Get the direction of the light.
-
inline void setConstantAttenuation( float constant_attenuation )
- Set the constant attenuation of the light.
-
inline float getConstantAttenuation() const
- Get the constant attenuation of the light.
-
inline void setLinearAttenuation( float linear_attenuation )
- Set the linear attenuation of the light.
-
inline float getLinearAttenuation() const
- Get the linear attenuation of the light.
-
inline void setQuadraticAttenuation( float quadratic_attenuation )
- Set the quadratic attenuation of the light.
-
inline float getQuadraticAttenuation() const
- Get the quadratic attenuation of the light.
-
inline void setSpotExponent( float spot_exponent )
- Set the spot exponent of the light.
-
inline float getSpotExponent() const
- Get the spot exponent of the light.
-
inline void setSpotCutoff( float spot_cutoff )
- Set the spot cutoff of the light.
-
inline float getSpotCutoff() const
- Get the spot cutoff of the light.
-
void captureLightState()
- Capture the lighting settings of the current OpenGL state and store them in this object
-
virtual void apply(State& state) const
- Apply the light's state to the OpenGL state machine.
Protected Fields-
int _lightnum
-
Vec4 _ambient
-
Vec4 _diffuse
-
Vec4 _specular
-
Vec4 _position
-
Vec3 _direction
-
float _constant_attenuation
-
float _linear_attenuation
-
float _quadratic_attenuation
-
float _spot_exponent
-
float _spot_cutoff
Protected Methods-
virtual ~Light()
-
void init()
- Initialize the light's settings with some decent defaults.
Public Methods-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp&) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual Type getType() const
-
virtual bool isTextureAttribute() const
-
bool operator < (const StateAttribute& rhs) const
-
bool operator == (const StateAttribute& rhs) const
-
bool operator != (const StateAttribute& rhs) const
-
virtual void compile(State&) const
Public Members-
typedef GLenum GLMode
-
typedef unsigned int GLModeValue
-
typedef unsigned int OverrideValue
-
enum Values
-
typedef unsigned int Type
-
enum Types
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Light state class which encapsulates OpenGL glLight() functionality
Light()
Light(const Light& light, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
META_StateAttribute(osg, Light, (Type)(LIGHT_0+_lightnum))
virtual int compare(const StateAttribute& sa) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
virtual void getAssociatedModes(std::vector<GLMode>& modes) const
void setLightNum(int num)
- Set which OpenGL light to operate on
int getLightNum() const
- Get which OpenGL light this osg::Light operates on
inline void setAmbient( const Vec4& ambient )
- Set the ambient component of the light.
inline const Vec4& getAmbient() const
- Get the ambient component of the light.
inline void setDiffuse( const Vec4& diffuse )
- Set the diffuse component of the light.
inline const Vec4& getDiffuse() const
- Get the diffuse component of the light.
inline void setSpecular( const Vec4& specular )
- Set the specular component of the light.
inline const Vec4& getSpecular() const
- Get the specular component of the light.
inline void setPosition( const Vec4& position )
- Set the position of the light.
inline const Vec4& getPosition() const
- Get the position of the light.
inline void setDirection( const Vec3& direction )
- Set the direction of the light.
inline const Vec3& getDirection() const
- Get the direction of the light.
inline void setConstantAttenuation( float constant_attenuation )
- Set the constant attenuation of the light.
inline float getConstantAttenuation() const
- Get the constant attenuation of the light.
inline void setLinearAttenuation( float linear_attenuation )
- Set the linear attenuation of the light.
inline float getLinearAttenuation() const
- Get the linear attenuation of the light.
inline void setQuadraticAttenuation( float quadratic_attenuation )
- Set the quadratic attenuation of the light.
inline float getQuadraticAttenuation() const
- Get the quadratic attenuation of the light.
inline void setSpotExponent( float spot_exponent )
- Set the spot exponent of the light.
inline float getSpotExponent() const
- Get the spot exponent of the light.
inline void setSpotCutoff( float spot_cutoff )
- Set the spot cutoff of the light.
inline float getSpotCutoff() const
- Get the spot cutoff of the light.
void captureLightState()
-
Capture the lighting settings of the current OpenGL state
and store them in this object
virtual void apply(State& state) const
- Apply the light's state to the OpenGL state machine.
virtual ~Light()
void init()
- Initialize the light's settings with some decent defaults.
int _lightnum
Vec4 _ambient
Vec4 _diffuse
Vec4 _specular
Vec4 _position
Vec3 _direction
float _constant_attenuation
float _linear_attenuation
float _quadratic_attenuation
float _spot_exponent
float _spot_cutoff
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|