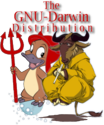
|
Base class for state attribuets
Inheritance:
Public Methods-
StateAttribute()
-
StateAttribute(const StateAttribute& sa, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
-
virtual Object* cloneType() const = 0
- Clone the type of an attribute, with Object* return type.
-
virtual Object* clone(const CopyOp&) const = 0
- Clone an attribute, with Object* return type.
-
virtual bool isSameKindAs(const Object* obj) const
- return true if this and obj are of the same kind of object
-
virtual const char* libraryName() const
- return the name of the attribute's library
-
virtual const char* className() const
- return the name of the attribute's class type
-
virtual Type getType() const = 0
- return the Type identifier of the attribute's class type
-
virtual bool isTextureAttribute() const
- return true if StateAttribute is a type which controls texturing and needs to be issued wrt to specific texture unit
-
virtual int compare(const StateAttribute& sa) const = 0
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
-
bool operator < (const StateAttribute& rhs) const
-
bool operator == (const StateAttribute& rhs) const
-
bool operator != (const StateAttribute& rhs) const
-
virtual void getAssociatedModes(std::vector<GLMode>& ) const
- return the modes associated with this StateSet
-
virtual void apply(State&) const = 0
- apply the OpenGL state attributes.
-
virtual void compile(State&) const
- default to nothing to compile - all state is applied immediately.
Public Members-
typedef GLenum GLMode
- GLMode is the value used in glEnable/glDisable(mode)
-
typedef unsigned int GLModeValue
- GLModeValue is used to specified whether an mode is enabled (ON) or disabled (OFF).
-
typedef unsigned int OverrideValue
- Override is used to specified the override behavior of StateAttributes from from parent to children.
-
enum Values
- list values which can be used in to set either GLModeValues or OverrideValues.
-
typedef unsigned int Type
- Type identifier to differentiate between different state types.
-
enum Types
- Values of StateAttribute::Type used to aid identification of different StateAttribute subclasses.
Protected Methods-
virtual ~StateAttribute()
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Base class for state attribuets
typedef GLenum GLMode
- GLMode is the value used in glEnable/glDisable(mode)
typedef unsigned int GLModeValue
- GLModeValue is used to specified whether an mode is enabled (ON) or disabled (OFF).
GLMoveValue is also used to specify the override behavior of modes from parent to children.
See enum Value description for more details.
typedef unsigned int OverrideValue
- Override is used to specified the override behavior of StateAttributes
from from parent to children.
See enum Value description for more details.
enum Values
- list values which can be used in to set either GLModeValues
or OverrideValues. When using in conjunction with GLModeValues
all Values have meaning. When using in conjection with
StateAttribute OverrideValue only OFF,OVERRIDE and INHERIT
are meaningful. However, they are useful when using GLModeValue
and OverrideValue in conjunction with each other as when using
StateSet::setAttributeAndModes(..).
OFF
- means that associated GLMode and Override is disabled
ON
- means that associated GLMode is enabled and Override is disabled
OVERRIDE
- Overriding of GLMode's or StateAttributes is enabled, so that state below it is overriden
PROTECTED
- Protecting of GLMode's os StateAttributes is enabled, so that state from above connot override this and below state
INHERIT
- means that GLMode or StateAttribute should in inherited from above
typedef unsigned int Type
- Type identifier to differentiate between different state types.
enum Types
- Values of StateAttribute::Type used to aid identification
of different StateAttribute subclasses. Each subclass defines
it own value in the virtual Type getType() method. When
extending the osg's StateAttribute's simply define your
own Type value which is unique, using the StateAttribute::Type
enum as a guide of what values to use. If your new subclass
needs to override a standard StateAttriubte then simple use
that types value.
TEXTURE
POLYGONMODE
POLYGONOFFSET
MATERIAL
ALPHAFUNC
ANTIALIAS
COLORTABLE
CULLFACE
FOG
FRONTFACE
LIGHT
LIGHT_0
LIGHT_1
LIGHT_2
LIGHT_3
LIGHT_4
LIGHT_5
LIGHT_6
LIGHT_7
POINT
LINEWIDTH
LINESTIPPLE
POLYGONSTIPPLE
SHADEMODEL
TEXENV
TEXGEN
TEXMAT
LIGHTMODEL
BLENDFUNC
STENCIL
COLORMASK
DEPTH
VIEWPORT
CLIPPLANE
CLIPPLANE_0
CLIPPLANE_1
CLIPPLANE_2
CLIPPLANE_3
CLIPPLANE_4
CLIPPLANE_5
COLORMATRIX
VERTEXPROGRAM
FRAGMENTPROGRAM
StateAttribute()
StateAttribute(const StateAttribute& sa, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
virtual Object* cloneType() const = 0
- Clone the type of an attribute, with Object* return type.
Must be defined by derived classes.
virtual Object* clone(const CopyOp&) const = 0
- Clone an attribute, with Object* return type.
Must be defined by derived classes.
virtual bool isSameKindAs(const Object* obj) const
- return true if this and obj are of the same kind of object
virtual const char* libraryName() const
- return the name of the attribute's library
virtual const char* className() const
- return the name of the attribute's class type
virtual Type getType() const = 0
- return the Type identifier of the attribute's class type
virtual bool isTextureAttribute() const
- return true if StateAttribute is a type which controls texturing and needs to be issued wrt to specific texture unit
virtual int compare(const StateAttribute& sa) const = 0
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
bool operator < (const StateAttribute& rhs) const
bool operator == (const StateAttribute& rhs) const
bool operator != (const StateAttribute& rhs) const
virtual void getAssociatedModes(std::vector<GLMode>& ) const
- return the modes associated with this StateSet
virtual void apply(State&) const = 0
- apply the OpenGL state attributes.
The global state for the current OpenGL context is passed
in to allow the StateAttribute to obtain details on the
the current context and state.
virtual void compile(State&) const
- default to nothing to compile - all state is applied immediately.
virtual ~StateAttribute()
- Direct child classes:
- Viewport
VertexProgram
Texture
TexMat
TexGen
TexEnvCombine
TexEnv
Stencil
ShadeModel
PolygonStipple
PolygonOffset
PolygonMode
Point
Material
LineWidth
LineStipple
LightModel
Light
FrontFace
Fog
Depth
CullFace
ColorMatrix
ColorMask
ClipPlane
BlendFunc
AlphaFunc
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|