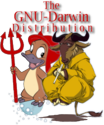
|
A quaternion class.
Public Fields-
Vec4 _fv
Public Methods-
inline Quat()
-
inline Quat( float x, float y, float z, float w )
-
inline Quat( const Vec4& v )
-
inline Quat( float angle, const Vec3& axis)
-
inline Quat( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
inline Vec4& asVec4()
-
inline const Vec4& asVec4() const
-
inline const Vec3 asVec3() const
-
inline void set(float x, float y, float z, float w)
-
inline void set(const osg::Vec4& v)
-
inline float& operator [] (int i)
-
inline float operator [] (int i) const
-
inline float& x()
-
inline float& y()
-
inline float& z()
-
inline float& w()
-
inline float x() const
-
inline float y() const
-
inline float z() const
-
inline float w() const
-
bool zeroRotation() const
- return true if the Quat represents a zero rotation, and therefore can be ignored in computations
-
inline const Quat operator * (float rhs) const
- Multiply by scalar
-
inline Quat& operator *= (float rhs)
- Unary multiply by scalar
-
inline const Quat operator*(const Quat& rhs) const
- Binary multiply
-
inline Quat& operator*=(const Quat& rhs)
- Unary multiply
-
inline const Quat operator / (float rhs) const
- Divide by scalar
-
inline Quat& operator /= (float rhs)
- Unary divide by scalar
-
inline const Quat operator/(const Quat& denom) const
- Binary divide
-
inline Quat& operator/=(const Quat& denom)
- Unary divide
-
inline const Quat operator + (const Quat& rhs) const
- Binary addition
-
inline Quat& operator += (const Quat& rhs)
- Unary addition
-
inline const Quat operator - (const Quat& rhs) const
- Binary subtraction
-
inline Quat& operator -= (const Quat& rhs)
- Unary subtraction
-
inline const Quat operator - () const
- Negation operator - returns the negative of the quaternion.
-
float length() const
- Length of the quaternion = sqrt( vec .
-
float length2() const
- Length of the quaternion = vec .
-
inline Quat conj() const
- Conjugate
-
inline const Quat inverse() const
- Multiplicative inverse method: q^(-1) = q^*/(qq^*)
-
void makeRotate( float angle, float x, float y, float z )
-
void makeRotate( float angle, const Vec3& vec )
-
void makeRotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
void makeRotate( const Vec3& vec1, const Vec3& vec2 )
- Make a rotation Quat which will rotate vec1 to vec2.
-
void getRotate( float& angle, float& x, float& y, float& z ) const
- Return the angle and vector components represented by the quaternion
-
void getRotate( float& angle, Vec3& vec ) const
- Return the angle and vector represented by the quaternion
-
void slerp( float t, const Quat& from, const Quat& to)
- Spherical Linear Interpolation.
-
void set( const Matrix& m )
- Set quaternion to be equivalent to specified matrix
-
void get( Matrix& m ) const
- Get the equivalent matrix for this quaternion
-
Matrix getMatrix() const
- Get the equivalent matrix for this quaternion
Documentation
A quaternion class. It can be used to represent an orientation in 3D space.
Vec4 _fv
inline Quat()
inline Quat( float x, float y, float z, float w )
inline Quat( const Vec4& v )
inline Quat( float angle, const Vec3& axis)
inline Quat( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
inline Vec4& asVec4()
inline const Vec4& asVec4() const
inline const Vec3 asVec3() const
inline void set(float x, float y, float z, float w)
inline void set(const osg::Vec4& v)
inline float& operator [] (int i)
inline float operator [] (int i) const
inline float& x()
inline float& y()
inline float& z()
inline float& w()
inline float x() const
inline float y() const
inline float z() const
inline float w() const
bool zeroRotation() const
- return true if the Quat represents a zero rotation, and therefore can be ignored in computations
inline const Quat operator * (float rhs) const
- Multiply by scalar
inline Quat& operator *= (float rhs)
- Unary multiply by scalar
inline const Quat operator*(const Quat& rhs) const
- Binary multiply
inline Quat& operator*=(const Quat& rhs)
- Unary multiply
inline const Quat operator / (float rhs) const
- Divide by scalar
inline Quat& operator /= (float rhs)
- Unary divide by scalar
inline const Quat operator/(const Quat& denom) const
- Binary divide
inline Quat& operator/=(const Quat& denom)
- Unary divide
inline const Quat operator + (const Quat& rhs) const
- Binary addition
inline Quat& operator += (const Quat& rhs)
- Unary addition
inline const Quat operator - (const Quat& rhs) const
- Binary subtraction
inline Quat& operator -= (const Quat& rhs)
- Unary subtraction
inline const Quat operator - () const
- Negation operator - returns the negative of the quaternion.
Basically just calls operator - () on the Vec4
float length() const
- Length of the quaternion = sqrt( vec . vec )
float length2() const
- Length of the quaternion = vec . vec
inline Quat conj() const
- Conjugate
inline const Quat inverse() const
- Multiplicative inverse method: q^(-1) = q^*/(qq^*)
void makeRotate( float angle, float x, float y, float z )
void makeRotate( float angle, const Vec3& vec )
void makeRotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
void makeRotate( const Vec3& vec1, const Vec3& vec2 )
- Make a rotation Quat which will rotate vec1 to vec2.
Generally take adot product to get the angle between these
and then use a cross product to get the rotation axis
Watch out for the two special cases of when the vectors
are co-incident or opposite in direction.
void getRotate( float& angle, float& x, float& y, float& z ) const
- Return the angle and vector components represented by the quaternion
void getRotate( float& angle, Vec3& vec ) const
- Return the angle and vector represented by the quaternion
void slerp( float t, const Quat& from, const Quat& to)
- Spherical Linear Interpolation.
As t goes from 0 to 1, the Quat object goes from "from" to "to".
void set( const Matrix& m )
- Set quaternion to be equivalent to specified matrix
void get( Matrix& m ) const
- Get the equivalent matrix for this quaternion
Matrix getMatrix() const
- Get the equivalent matrix for this quaternion
- This class has no child classes.
- Friends:
- inline std::ostream& operator << (std::ostream& output, const Quat& vec)
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|