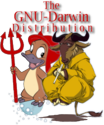
|
An ImposterSprite is a textured quad which is rendered in place a 3D geometry.
Inheritance:
Public Fields-
Vec4 _color
Public Methods-
ImpostorSprite()
-
virtual Object* cloneType() const
- Clone an object of the same type as an ImpostorSprite
-
virtual Object* clone(const CopyOp&) const
- Clone on ImpostorSprite just returns a clone of type, since it is not appropriate to share data of an ImpostorSprite
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
void setParent(Impostor* parent)
- Set the parent, which must be an Impostor.
-
Impostor* getParent()
- Get the parent, which is an Impostor.
-
const Impostor* getParent() const
- Get the const parent, which is an Impostor.
-
inline void setStoredLocalEyePoint(const Vec3& v)
- Set the eye point for when the ImpsotorSprite was snapped
-
inline const Vec3& getStoredLocalEyePoint() const
- Get the eye point for when the ImpsotorSprite was snapped
-
inline void setLastFrameUsed(int frameNumber)
- Set the frame number for when the ImpostorSprite was last used in rendering
-
inline int getLastFrameUsed() const
- Get the frame number for when the ImpostorSprite was last used in rendering
-
inline Vec3* getCoords()
- Get the coordinates of the corners of the quad.
-
inline const Vec3* getCoords() const
- Get the const coordinates of the corners of the quad
-
inline Vec2* getTexCoords()
- Get the texture coordinates of the corners of the quad.
-
inline const Vec2* getTexCoords() const
- Get the const texture coordinates of the corners of the quad
-
inline Vec3* getControlCoords()
- Get the control coordinates of the corners of the quad.
-
inline const Vec3* getControlCoords() const
- Get the const control coordinates of the corners of the quad
-
float calcPixelError(const Matrix& MVPW) const
- calculate the pixel error value for passing in the ModelViewProjectionWindow transform, which transform local coords into screen space
-
void setTexture(Texture2D* tex, int s, int t)
-
Texture2D* getTexture()
-
const Texture2D* getTexture() const
-
int s() const
-
int t() const
-
virtual void drawImplementation(State& state) const
- draw ImpostorSprite directly.
-
virtual bool supports(AttributeFunctor&) const
- return true, osg::ImpostorSprite does support accept(AttributeFunctor&)
-
virtual void accept(AttributeFunctor& af)
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has
-
virtual bool supports(ConstAttributeFunctor&) const
- return true, osg::ImpostorSprite does support accept(ConstAttributeFunctor&)
-
virtual void accept(ConstAttributeFunctor& af) const
- accept an ConstAttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has
-
virtual bool supports(PrimitiveFunctor&) const
- return true, osg::ImpostorSprite does support accept(PrimitiveFunctor&)
-
virtual void accept(PrimitiveFunctor& pf) const
- accept a PrimtiveFunctor and call its methods to tell it about the interal primtives that this Drawable has
Protected Fields-
Impostor* _parent
-
ImpostorSpriteManager* _ism
-
ImpostorSprite* _previous
-
ImpostorSprite* _next
-
int _lastFrameUsed
-
Vec3 _storedLocalEyePoint
-
Vec3 _coords[4]
-
Vec2 _texcoords[4]
-
Vec3 _controlcoords[4]
-
Texture2D* _texture
-
int _s
-
int _t
Protected Methods-
ImpostorSprite(const ImpostorSprite&)
-
ImpostorSprite& operator = (const ImpostorSprite&)
-
virtual ~ImpostorSprite()
-
virtual bool computeBound() const
Public Methods-
virtual Geometry* asGeometry()
-
virtual const Geometry* asGeometry() const
-
inline const ParentList& getParents() const
-
inline ParentList getParents()
-
inline unsigned int getNumParents() const
-
inline void setStateSet(StateSet* state)
-
inline StateSet* getStateSet()
-
inline const StateSet* getStateSet() const
-
StateSet* getOrCreateStateSet()
-
void dirtyBound()
-
inline const BoundingBox& getBound() const
-
inline void setShape(Shape* shape)
-
inline Shape* getShape()
-
inline const Shape* getShape() const
-
void setSupportsDisplayList(bool flag)
-
inline bool getSupportsDisplayList() const
-
void setUseDisplayList(bool flag)
-
inline bool getUseDisplayList() const
-
void dirtyDisplayList()
-
virtual void compile(State& state) const
-
void setUpdateCallback(UpdateCallback* ac)
-
UpdateCallback* getUpdateCallback()
-
void setAppCallback(AppCallback* ac)
-
AppCallback* getAppCallback()
-
const AppCallback* getAppCallback() const
-
void setCullCallback(CullCallback* cc)
-
CullCallback* getCullCallback()
-
const CullCallback* getCullCallback() const
-
void setDrawCallback(DrawCallback* dc)
-
DrawCallback* getDrawCallback()
-
const DrawCallback* getDrawCallback() const
-
static void deleteDisplayList(uint contextID, uint globj)
-
static void flushDeletedDisplayLists(uint contextID)
-
inline void draw(State& state) const
Public Members-
typedef std::vector<Node*> ParentList
-
struct UpdateCallback: public virtual osg::Referenced
-
struct AppCallback: public UpdateCallback
-
struct CullCallback: public virtual osg::Referenced
-
struct DrawCallback: public virtual osg::Referenced
-
enum AttributeType
-
class AttributeFunctor
-
class ConstAttributeFunctor
-
class PrimitiveFunctor
Protected Fields-
ParentList _parents
-
ref_ptr<StateSet> _stateset
-
mutable BoundingBox _bbox
-
mutable bool _bbox_computed
-
ref_ptr<Shape> _shape
-
bool _supportsDisplayList
-
bool _useDisplayList
-
mutable GLObjectList _globjList
-
ref_ptr<UpdateCallback> _updateCallback
-
ref_ptr<DrawCallback> _drawCallback
-
ref_ptr<CullCallback> _cullCallback
Protected Methods-
void addParent(osg::Node* node)
-
void removeParent(osg::Node* node)
Protected Members-
typedef osg::buffered_value<uint> GLObjectList
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
An ImposterSprite is a textured quad which is rendered in place a
3D geometry. The ImposterSprite is generated by rendering the original
3D geometry to a texture as an image cache. The ImpostorSprite is
automatically generated by the osgUtil::CullVisitor so it not
necessary to deal with it directly.
ImpostorSprite()
virtual Object* cloneType() const
- Clone an object of the same type as an ImpostorSprite
virtual Object* clone(const CopyOp&) const
- Clone on ImpostorSprite just returns a clone of type,
since it is not appropriate to share data of an ImpostorSprite
virtual bool isSameKindAs(const Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
void setParent(Impostor* parent)
- Set the parent, which must be an Impostor.
Unlike conventional Drawables, ImpostorSprite's can only ever have
one parent.
Impostor* getParent()
- Get the parent, which is an Impostor.
const Impostor* getParent() const
- Get the const parent, which is an Impostor.
inline void setStoredLocalEyePoint(const Vec3& v)
- Set the eye point for when the ImpsotorSprite was snapped
inline const Vec3& getStoredLocalEyePoint() const
- Get the eye point for when the ImpsotorSprite was snapped
inline void setLastFrameUsed(int frameNumber)
- Set the frame number for when the ImpostorSprite was last used in rendering
inline int getLastFrameUsed() const
- Get the frame number for when the ImpostorSprite was last used in rendering
inline Vec3* getCoords()
- Get the coordinates of the corners of the quad.
Stored in the order, [0] - top_left, [1] - bottom_left, [2] - bottom_right, [3] - top_left.
inline const Vec3* getCoords() const
- Get the const coordinates of the corners of the quad
inline Vec2* getTexCoords()
- Get the texture coordinates of the corners of the quad.
Stored in the order, [0] - top_left, [1] - bottom_left, [2] - bottom_right, [3] - top_left.
inline const Vec2* getTexCoords() const
- Get the const texture coordinates of the corners of the quad
inline Vec3* getControlCoords()
- Get the control coordinates of the corners of the quad.
The control coordinates are the corners of the quad projected
out onto the front face of bounding box which enclosed the impostor
geometry when it was pre-rendered into the impostor sprite's texture.
At the point of creation/or update of the impostor sprite the control
coords will lie on top of the corners of the quad in screen space - with a pixel error
or zero. Once the camera moves relative to the impostor sprite the
control coords will no longer lie on top of the corners of the quad in
screen space - a pixel error will have accumulated. This pixel error
can then be used to determine whether the impostor needs to be updated.
Stored in the order, [0] - top_left, [1] - bottom_left, [2] - bottom_right, [3] - top_left.
inline const Vec3* getControlCoords() const
- Get the const control coordinates of the corners of the quad
float calcPixelError(const Matrix& MVPW) const
- calculate the pixel error value for passing in the ModelViewProjectionWindow transform,
which transform local coords into screen space
void setTexture(Texture2D* tex, int s, int t)
Texture2D* getTexture()
const Texture2D* getTexture() const
int s() const
int t() const
virtual void drawImplementation(State& state) const
- draw ImpostorSprite directly.
virtual bool supports(AttributeFunctor&) const
- return true, osg::ImpostorSprite does support accept(AttributeFunctor&)
virtual void accept(AttributeFunctor& af)
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has
virtual bool supports(ConstAttributeFunctor&) const
- return true, osg::ImpostorSprite does support accept(ConstAttributeFunctor&)
virtual void accept(ConstAttributeFunctor& af) const
- accept an ConstAttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has
virtual bool supports(PrimitiveFunctor&) const
- return true, osg::ImpostorSprite does support accept(PrimitiveFunctor&)
virtual void accept(PrimitiveFunctor& pf) const
- accept a PrimtiveFunctor and call its methods to tell it about the interal primtives that this Drawable has
Vec4 _color
ImpostorSprite(const ImpostorSprite&)
ImpostorSprite& operator = (const ImpostorSprite&)
virtual ~ImpostorSprite()
virtual bool computeBound() const
Impostor* _parent
ImpostorSpriteManager* _ism
ImpostorSprite* _previous
ImpostorSprite* _next
int _lastFrameUsed
Vec3 _storedLocalEyePoint
Vec3 _coords[4]
Vec2 _texcoords[4]
Vec3 _controlcoords[4]
Texture2D* _texture
int _s
int _t
- This class has no child classes.
- Friends:
- class osg::ImpostorSpriteManager
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|