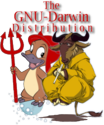
|
Encapsulates OpenGL state modes and attributes.
Inheritance:
Public Methods-
StateSet()
-
StateSet(const StateSet&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp& copyop) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
int compare(const StateSet& rhs, bool compareAttributeContents=false) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
-
bool operator < (const StateSet& rhs) const
-
bool operator == (const StateSet& rhs) const
-
bool operator != (const StateSet& rhs) const
-
void setGlobalDefaults()
- set all the modes to on or off so that it defines a complete state, typically used for a default global state
-
void setAllToInherit()
- set all the modes to inherit, typically used to signify nodes which inherit all of their modes for the global state
-
void merge(const StateSet& rhs)
- merge this stateset with stateset rhs, this overrides the rhs if OVERRIDE is specified, otherwise rhs takes precedence
-
void setMode(StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
- set this StateSet to contain specified GLMode and value
-
void setModeToInherit(StateAttribute::GLMode mode)
- set this StateSet to inherit specified GLMode type from parents.
-
StateAttribute::GLModeValue getMode(StateAttribute::GLMode mode) const
- get specified GLModeValue for specified GLMode.
-
inline ModeList& getModeList()
- return the list of all GLModes contained in this StateSet
-
inline const ModeList& getModeList() const
- return the const list of all GLModes contained in this const StateSet
-
void setAttribute(StateAttribute* attribute, StateAttribute::OverrideValue value=StateAttribute::OFF)
- set this StateSet to contain specified attribute and override flag
-
void setAttributeAndModes(StateAttribute* attribute, StateAttribute::GLModeValue value=StateAttribute::ON)
- set this StateSet to contain specified attribute and set the associated GLMode's to specified value
-
void setAttributeToInherit(StateAttribute::Type type)
- set this StateSet to inherit specified attribute type from parents.
-
StateAttribute* getAttribute(StateAttribute::Type type)
- get specified StateAttribute for specified type.
-
const StateAttribute* getAttribute(StateAttribute::Type type) const
- get specified const StateAttribute for specified type.
-
const RefAttributePair* getAttributePair(StateAttribute::Type type) const
- get specified RefAttributePair for specified type.
-
inline AttributeList& getAttributeList()
- return the list of all StateAttributes contained in this StateSet
-
inline const AttributeList& getAttributeList() const
- return the const list of all StateAttributes contained in this const StateSet
-
void setTextureMode(unsigned int unit, StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
- set this StateSet to contain specified GLMode and value
-
void setTextureModeToInherit(unsigned int unit, StateAttribute::GLMode mode)
- set this StateSet to inherit specified GLMode type from parents.
-
StateAttribute::GLModeValue getTextureMode(unsigned int unit, StateAttribute::GLMode mode) const
- get specified GLModeValue for specified GLMode.
-
inline TextureModeList& getTextureModeList()
- return the list of all Texture related GLModes contained in this StateSet
-
inline const TextureModeList& getTextureModeList() const
- return the const list of all Texture related GLModes contained in this const StateSet
-
void setTextureAttribute(unsigned int unit, StateAttribute* attribute, StateAttribute::OverrideValue value=StateAttribute::OFF)
- set this StateSet to contain specified attribute and override flag
-
void setTextureAttributeAndModes(unsigned int unit, StateAttribute* attribute, StateAttribute::GLModeValue value=StateAttribute::ON)
- set this StateSet to contain specified attribute and set the associated GLMode's to specified value
-
void setTextureAttributeToInherit(unsigned int unit, StateAttribute::Type type)
- set this StateSet to inherit specified attribute type from parents.
-
StateAttribute* getTextureAttribute(unsigned int unit, StateAttribute::Type type)
- get specified Texture related StateAttribute for specified type.
-
const StateAttribute* getTextureAttribute(unsigned int unit, StateAttribute::Type type) const
- get specified Texture related const StateAttribute for specified type.
-
const RefAttributePair* getTextureAttributePair(unsigned int unit, StateAttribute::Type type) const
- get specified Texture related RefAttributePair for specified type.
-
inline TextureAttributeList& getTextureAttributeList()
- return the list of all Texture related StateAttributes contained in this StateSet
-
inline const TextureAttributeList& getTextureAttributeList() const
- return the const list of all Texture related StateAttributes contained in this const StateSet
-
void setAssociatedModes(const StateAttribute* attribute, StateAttribute::GLModeValue value)
-
void setAssociatedTextureModes(unsigned int unit, const StateAttribute* attribute, StateAttribute::GLModeValue value)
-
void setRenderingHint(int hint)
- set the RenderingHint of the StateSet.
-
inline int getRenderingHint() const
- get the RenderingHint of the StateSet
-
void setRenderBinDetails(int binNum, const std::string& binName, RenderBinMode mode=USE_RENDERBIN_DETAILS)
- set the render bin details
-
void setRenderBinToInherit()
- set the render bin details to inherit
-
inline RenderBinMode getRenderBinMode() const
- get the render bin mode
-
inline bool useRenderBinDetails() const
- get whether the render bin details are set and should be used
-
inline int getBinNumber() const
- get the render bin number
-
inline const std::string& getBinName() const
- get the render bin name
-
void compile(State& state) const
- call compile on all StateAttributes contained within this StateSet
Public Members-
typedef std::map<StateAttribute::GLMode,StateAttribute::GLModeValue> ModeList
- a container to map GLModes to their respective GLModeValues
-
typedef std::pair<ref_ptr<StateAttribute>,StateAttribute::OverrideValue> RefAttributePair
- simple pairing between an attribute and its override flag
-
typedef std::map<StateAttribute::Type,RefAttributePair> AttributeList
- a container to map StateAttribyte::Types to their respective RefAttributePair
-
typedef std::vector<ModeList> TextureModeList
-
typedef std::vector<AttributeList> TextureAttributeList
-
enum RenderingHint
-
enum RenderBinMode
Protected Fields-
ModeList _modeList
-
AttributeList _attributeList
-
TextureModeList _textureModeList
-
TextureAttributeList _textureAttributeList
-
int _renderingHint
-
RenderBinMode _binMode
-
int _binNum
-
std::string _binName
Protected Methods-
virtual ~StateSet()
-
StateSet& operator = (const StateSet&)
-
inline ModeList& getOrCreateTextureModeList(unsigned int unit)
-
inline AttributeList& getOrCreateTextureAttributeList(unsigned int unit)
-
int compareModes(const ModeList& lhs, const ModeList& rhs)
-
int compareAttributePtrs(const AttributeList& lhs, const AttributeList& rhs)
-
int compareAttributeContents(const AttributeList& lhs, const AttributeList& rhs)
-
void setMode(ModeList& modeList, StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
-
void setModeToInherit(ModeList& modeList, StateAttribute::GLMode mode)
-
StateAttribute::GLModeValue getMode(const ModeList& modeList, StateAttribute::GLMode mode) const
-
void setAssociatedModes(ModeList& modeList, const StateAttribute* attribute, StateAttribute::GLModeValue value)
-
void setAttribute(AttributeList& attributeList, StateAttribute* attribute, const StateAttribute::OverrideValue value=StateAttribute::OFF)
-
StateAttribute* getAttribute(AttributeList& attributeList, const StateAttribute::Type type)
-
const StateAttribute* getAttribute(const AttributeList& attributeList, const StateAttribute::Type type) const
-
const RefAttributePair* getAttributePair(const AttributeList& attributeList, const StateAttribute::Type type) const
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Encapsulates OpenGL state modes and attributes.
Used to specific textures etc of osg::Drawable's which hold references
to a single osg::StateSet. StateSet can be shared between Drawable's
and is recommend if possible as it minimize expensive state changes
in the graphics pipeline.
StateSet()
StateSet(const StateSet&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
virtual Object* cloneType() const
virtual Object* clone(const CopyOp& copyop) const
virtual bool isSameKindAs(const Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
int compare(const StateSet& rhs, bool compareAttributeContents=false) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
bool operator < (const StateSet& rhs) const
bool operator == (const StateSet& rhs) const
bool operator != (const StateSet& rhs) const
void setGlobalDefaults()
- set all the modes to on or off so that it defines a
complete state, typically used for a default global state
void setAllToInherit()
- set all the modes to inherit, typically used to signify
nodes which inherit all of their modes for the global state
void merge(const StateSet& rhs)
- merge this stateset with stateset rhs, this overrides
the rhs if OVERRIDE is specified, otherwise rhs takes precedence
typedef std::map<StateAttribute::GLMode,StateAttribute::GLModeValue> ModeList
- a container to map GLModes to their respective GLModeValues
void setMode(StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
- set this StateSet to contain specified GLMode and value
void setModeToInherit(StateAttribute::GLMode mode)
- set this StateSet to inherit specified GLMode type from parents.
has the effect of deleting any GlMode of specified type from StateSet.
StateAttribute::GLModeValue getMode(StateAttribute::GLMode mode) const
- get specified GLModeValue for specified GLMode.
returns INHERIT if no GLModeValue is contained within StateSet.
inline ModeList& getModeList()
- return the list of all GLModes contained in this StateSet
inline const ModeList& getModeList() const
- return the const list of all GLModes contained in this const StateSet
typedef std::pair<ref_ptr<StateAttribute>,StateAttribute::OverrideValue> RefAttributePair
- simple pairing between an attribute and its override flag
typedef std::map<StateAttribute::Type,RefAttributePair> AttributeList
- a container to map StateAttribyte::Types to their respective RefAttributePair
void setAttribute(StateAttribute* attribute, StateAttribute::OverrideValue value=StateAttribute::OFF)
- set this StateSet to contain specified attribute and override flag
void setAttributeAndModes(StateAttribute* attribute, StateAttribute::GLModeValue value=StateAttribute::ON)
- set this StateSet to contain specified attribute and set the associated GLMode's to specified value
void setAttributeToInherit(StateAttribute::Type type)
- set this StateSet to inherit specified attribute type from parents.
has the effect of deleting any state attributes of specified type from StateSet.
StateAttribute* getAttribute(StateAttribute::Type type)
- get specified StateAttribute for specified type.
returns NULL if no type is contained within StateSet.
const StateAttribute* getAttribute(StateAttribute::Type type) const
- get specified const StateAttribute for specified type.
returns NULL if no type is contained within const StateSet.
const RefAttributePair* getAttributePair(StateAttribute::Type type) const
- get specified RefAttributePair for specified type.
returns NULL if no type is contained within StateSet.
inline AttributeList& getAttributeList()
- return the list of all StateAttributes contained in this StateSet
inline const AttributeList& getAttributeList() const
- return the const list of all StateAttributes contained in this const StateSet
typedef std::vector<ModeList> TextureModeList
void setTextureMode(unsigned int unit, StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
- set this StateSet to contain specified GLMode and value
void setTextureModeToInherit(unsigned int unit, StateAttribute::GLMode mode)
- set this StateSet to inherit specified GLMode type from parents.
has the effect of deleting any GlMode of specified type from StateSet.
StateAttribute::GLModeValue getTextureMode(unsigned int unit, StateAttribute::GLMode mode) const
- get specified GLModeValue for specified GLMode.
returns INHERIT if no GLModeValue is contained within StateSet.
inline TextureModeList& getTextureModeList()
- return the list of all Texture related GLModes contained in this StateSet
inline const TextureModeList& getTextureModeList() const
- return the const list of all Texture related GLModes contained in this const StateSet
typedef std::vector<AttributeList> TextureAttributeList
void setTextureAttribute(unsigned int unit, StateAttribute* attribute, StateAttribute::OverrideValue value=StateAttribute::OFF)
- set this StateSet to contain specified attribute and override flag
void setTextureAttributeAndModes(unsigned int unit, StateAttribute* attribute, StateAttribute::GLModeValue value=StateAttribute::ON)
- set this StateSet to contain specified attribute and set the associated GLMode's to specified value
void setTextureAttributeToInherit(unsigned int unit, StateAttribute::Type type)
- set this StateSet to inherit specified attribute type from parents.
has the effect of deleting any state attributes of specified type from StateSet.
StateAttribute* getTextureAttribute(unsigned int unit, StateAttribute::Type type)
- get specified Texture related StateAttribute for specified type.
returns NULL if no type is contained within StateSet.
const StateAttribute* getTextureAttribute(unsigned int unit, StateAttribute::Type type) const
- get specified Texture related const StateAttribute for specified type.
returns NULL if no type is contained within const StateSet.
const RefAttributePair* getTextureAttributePair(unsigned int unit, StateAttribute::Type type) const
- get specified Texture related RefAttributePair for specified type.
returns NULL if no type is contained within StateSet.
inline TextureAttributeList& getTextureAttributeList()
- return the list of all Texture related StateAttributes contained in this StateSet
inline const TextureAttributeList& getTextureAttributeList() const
- return the const list of all Texture related StateAttributes contained in this const StateSet
void setAssociatedModes(const StateAttribute* attribute, StateAttribute::GLModeValue value)
void setAssociatedTextureModes(unsigned int unit, const StateAttribute* attribute, StateAttribute::GLModeValue value)
enum RenderingHint
DEFAULT_BIN
OPAQUE_BIN
TRANSPARENT_BIN
void setRenderingHint(int hint)
- set the RenderingHint of the StateSet.
RenderingHint is used by osgUtil::Renderer to determine which
draw bin to drop associated osg::Drawables in. For opaque
objects OPAQUE_BIN would typical used, which TRANSPARENT_BIN
should be used for objects which need to be depth sorted.
inline int getRenderingHint() const
- get the RenderingHint of the StateSet
enum RenderBinMode
INHERIT_RENDERBIN_DETAILS
USE_RENDERBIN_DETAILS
OVERRIDE_RENDERBIN_DETAILS
ENCLOSE_RENDERBIN_DETAILS
void setRenderBinDetails(int binNum, const std::string& binName, RenderBinMode mode=USE_RENDERBIN_DETAILS)
- set the render bin details
void setRenderBinToInherit()
- set the render bin details to inherit
inline RenderBinMode getRenderBinMode() const
- get the render bin mode
inline bool useRenderBinDetails() const
- get whether the render bin details are set and should be used
inline int getBinNumber() const
- get the render bin number
inline const std::string& getBinName() const
- get the render bin name
void compile(State& state) const
- call compile on all StateAttributes contained within this StateSet
virtual ~StateSet()
StateSet& operator = (const StateSet&)
ModeList _modeList
AttributeList _attributeList
TextureModeList _textureModeList
TextureAttributeList _textureAttributeList
inline ModeList& getOrCreateTextureModeList(unsigned int unit)
inline AttributeList& getOrCreateTextureAttributeList(unsigned int unit)
int compareModes(const ModeList& lhs, const ModeList& rhs)
int compareAttributePtrs(const AttributeList& lhs, const AttributeList& rhs)
int compareAttributeContents(const AttributeList& lhs, const AttributeList& rhs)
void setMode(ModeList& modeList, StateAttribute::GLMode mode, StateAttribute::GLModeValue value)
void setModeToInherit(ModeList& modeList, StateAttribute::GLMode mode)
StateAttribute::GLModeValue getMode(const ModeList& modeList, StateAttribute::GLMode mode) const
void setAssociatedModes(ModeList& modeList, const StateAttribute* attribute, StateAttribute::GLModeValue value)
void setAttribute(AttributeList& attributeList, StateAttribute* attribute, const StateAttribute::OverrideValue value=StateAttribute::OFF)
StateAttribute* getAttribute(AttributeList& attributeList, const StateAttribute::Type type)
const StateAttribute* getAttribute(const AttributeList& attributeList, const StateAttribute::Type type) const
const RefAttributePair* getAttributePair(const AttributeList& attributeList, const StateAttribute::Type type) const
int _renderingHint
RenderBinMode _binMode
int _binNum
std::string _binName
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|