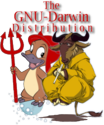
|
Camera class for encapsulating the view position and orientation and projection (lens) used.
Inheritance:
Public Methods-
Camera(DisplaySettings* ds=NULL)
-
Camera(const Camera&)
-
Camera& operator=(const Camera&)
-
ProjectionType getProjectionType() const
- Get the projection type set by setOtho,setOtho2D,setFrustum, and set perspective methods
-
void setOrtho(double left, double right, double bottom, double top, double zNear, double zFar)
- Set a orthographic projection.
-
void setOrtho2D(double left, double right, double bottom, double top)
- Set a 2D orthographic projection.
-
void setFrustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Set a perspective projection.
-
void setPerspective(double fovy, double aspectRatio, double zNear, double zFar)
- Set a symmetrical perspective projection, See gluPerspective for further details.
-
void setFOV(double fovx, double fovy, double zNear, double zFar)
- Set a sysmmetical perspective projection using field of view
-
void setNearFar(double zNear, double zFar)
- Set the near and far clipping planes
-
void setAdjustAspectRatioMode(AdjustAspectRatioMode aam)
- Set the way that the vertical or horizontal dimensions of the window are adjusted on a resize.
-
AdjustAspectRatioMode getAdjustAspectRatioMode() const
- Get the way that the vertical or horizontal dimensions of the window are adjusted on a resize.
-
void adjustAspectRatio(double newAspectRatio)
- Adjust the clipping planes to account for a new window aspect ratio.
-
void adjustAspectRatio(double newAspectRatio, AdjustAspectRatioMode aa)
- Adjust the clipping planes to account for a new window aspect ratio.
-
double left() const
-
double right() const
-
double bottom() const
-
double top() const
-
double zNear() const
-
double zFar() const
-
double calc_fovy() const
- Calculate and return the equivalent fovx for the current project setting.
-
double calc_fovx() const
- Calculate and return the equivalent fovy for the current project setting.
-
double calc_aspectRatio() const
- Calculate and return the projection aspect ratio.
-
LookAtType getLookAtType() const
-
void home()
- hardwired home view for now, looking straight down the Z axis at the origin, with 'up' being the y axis
-
void setView(const Vec3& eyePoint, const Vec3& lookPoint, const Vec3& upVector)
- Set the View, the up vector should be orthogonal to the look vector.
-
void setLookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- set the position and orientation of the camera, using the same convention as gluLookAt
-
void setLookAt(double eyeX, double eyeY, double eyeZ, double centerX, double centerY, double centerZ, double upX, double upY, double upZ)
- set the position and orientation of the camera, using the same convention as gluLookAt
-
void transformLookAt(const Matrix& matrix)
- post multiple the existing eye point and orientation by matrix.
-
void ensureOrthogonalUpVector()
-
inline const Vec3& getEyePoint() const
- get the eye point.
-
inline const Vec3& getCenterPoint() const
- get the center point.
-
inline const Vec3& getUpVector() const
- get the up vector
-
Vec3 getLookVector() const
- calculate look vector
-
Vec3 getSideVector() const
- calculate side vector
-
inline float getLookDistance() const
- calculate the look distance which is the distance between the eye and the center
-
void attachTransform(TransformMode mode, RefMatrix* modelTransform=0)
- Attach a transform matrix which is applied after the camera look at.
-
Matrix* getTransform(TransformMode mode)
-
const Matrix* getTransform(TransformMode mode) const
-
void setFusionDistanceMode(FusionDistanceMode mode)
- Set the mode of the fusion distance function which in use to calculate the fusion distance used in stereo rendering.
-
FusionDistanceMode getFusionDistanceMode() const
- Get the mode of the fusion distance function
-
void setFusionDistanceRatio(float ratio)
- Set the ratio of the fusion distance function which in use to calculate the fusion distance used in stereo rendering.
-
float getFusionDistanceRatio() const
- Get the ratio of the fusion distance function
-
float getFusionDistance() const
- Calculate and return the fusion distance, using the FusionDistanceFunction
-
void setScreenDistance(float screenDistance)
- Set the physical distance between the viewers eyes and the display system.
-
float getScreenDistance() const
- Get the physical distance between the viewers eyes and the display system
-
Matrix getProjectionMatrix() const
- Get the Projection Matrix
-
Matrix getModelViewMatrix() const
- Get the ModelView matrix.
-
inline Polytope getViewFrustum() const
- Get the camera view frustum
Public Members-
enum ProjectionType
- Range of projection types.
-
enum AdjustAspectRatioMode
- Use in combination with adjustAspectRatio, to control the change in frustum clipping planes to account for changes in windows aspect ratio,
-
enum LookAtType
-
enum TransformMode
-
enum FusionDistanceMode
Protected Fields-
ProjectionType _projectionType
-
AdjustAspectRatioMode _adjustAspectRatioMode
-
double _left
-
double _right
-
double _bottom
-
double _top
-
double _zNear
-
double _zFar
-
LookAtType _lookAtType
-
Vec3 _eye
-
Vec3 _center
-
Vec3 _up
-
TransformMode _attachedTransformMode
-
ref_ptr<RefMatrix> _eyeToModelTransform
-
ref_ptr<RefMatrix> _modelToEyeTransform
-
float _screenDistance
-
FusionDistanceMode _fusionDistanceMode
-
float _fusionDistanceRatio
Protected Methods-
virtual ~Camera()
-
void copy(const Camera&)
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Camera class for encapsulating the view position and orientation and
projection (lens) used. Creates a projection and modelview matrices
which can be used to set OpenGL's PROJECTION and MODELVIEW matrices
respectively.
Camera(DisplaySettings* ds=NULL)
Camera(const Camera&)
Camera& operator=(const Camera&)
enum ProjectionType
- Range of projection types.
ORTHO2D is a special case of ORTHO where the near and far planes
are equal to -1 and 1 respectively.
PERSPECTIVE is a special case of FRUSTUM where the left & right
and bottom and top and symmetrical.
ORTHO
ORTHO2D
FRUSTUM
PERSPECTIVE
ProjectionType getProjectionType() const
- Get the projection type set by setOtho,setOtho2D,setFrustum,
and set perspective methods
void setOrtho(double left, double right, double bottom, double top, double zNear, double zFar)
- Set a orthographic projection. See glOrtho for further details.
void setOrtho2D(double left, double right, double bottom, double top)
- Set a 2D orthographic projection. See gluOrtho2D for further details.
void setFrustum(double left, double right, double bottom, double top, double zNear, double zFar)
- Set a perspective projection. See glFrustum for further details.
void setPerspective(double fovy, double aspectRatio, double zNear, double zFar)
- Set a symmetrical perspective projection, See gluPerspective for further details.
Aspect ratio is defined as width/height.
void setFOV(double fovx, double fovy, double zNear, double zFar)
- Set a sysmmetical perspective projection using field of view
void setNearFar(double zNear, double zFar)
- Set the near and far clipping planes
enum AdjustAspectRatioMode
- Use in combination with adjustAspectRatio, to control
the change in frustum clipping planes to account for
changes in windows aspect ratio,
ADJUST_VERTICAL
ADJUST_HORIZONTAL
ADJUST_NONE
void setAdjustAspectRatioMode(AdjustAspectRatioMode aam)
- Set the way that the vertical or horizontal dimensions of the window
are adjusted on a resize.
AdjustAspectRatioMode getAdjustAspectRatioMode() const
- Get the way that the vertical or horizontal dimensions of the window
are adjusted on a resize.
void adjustAspectRatio(double newAspectRatio)
- Adjust the clipping planes to account for a new window aspect ratio.
Typically used after resizing a window. Aspect ratio is defined as
width/height.
void adjustAspectRatio(double newAspectRatio, AdjustAspectRatioMode aa)
- Adjust the clipping planes to account for a new window aspect ratio.
Typicall used after resizeing a window. Aspect ratio is defined as
width/height.
double left() const
double right() const
double bottom() const
double top() const
double zNear() const
double zFar() const
double calc_fovy() const
- Calculate and return the equivalent fovx for the current project setting.
This value is only valid for when a symmetric perspective projection exists.
i.e. getProjectionType()==PERSPECTIVE.
double calc_fovx() const
- Calculate and return the equivalent fovy for the current project setting.
This value is only valid for when a symmetric perspective projection exists.
i.e. getProjectionType()==PERSPECTIVE.
double calc_aspectRatio() const
- Calculate and return the projection aspect ratio.
Aspect ratio is defined as width/height.
enum LookAtType
USE_HOME_POSITION
USE_EYE_AND_QUATERNION
USE_EYE_CENTER_AND_UP
LookAtType getLookAtType() const
void home()
-
hardwired home view for now, looking straight down the
Z axis at the origin, with 'up' being the y axis
void setView(const Vec3& eyePoint, const Vec3& lookPoint, const Vec3& upVector)
-
Set the View, the up vector should be orthogonal to the look vector.
setView is now mapped to setLookAt(eye,center,up), and is only
kept for backwards compatibility.
void setLookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
- set the position and orientation of the camera, using the same convention as
gluLookAt
void setLookAt(double eyeX, double eyeY, double eyeZ, double centerX, double centerY, double centerZ, double upX, double upY, double upZ)
- set the position and orientation of the camera, using the same convention as
gluLookAt
void transformLookAt(const Matrix& matrix)
- post multiple the existing eye point and orientation by matrix.
note, does not affect any ModelTransforms that are applied.
void ensureOrthogonalUpVector()
inline const Vec3& getEyePoint() const
- get the eye point.
inline const Vec3& getCenterPoint() const
- get the center point.
inline const Vec3& getUpVector() const
- get the up vector
Vec3 getLookVector() const
- calculate look vector
Vec3 getSideVector() const
- calculate side vector
inline float getLookDistance() const
- calculate the look distance which is the distance between the eye and the center
enum TransformMode
EYE_TO_MODEL
MODEL_TO_EYE
NO_ATTACHED_TRANSFORM
void attachTransform(TransformMode mode, RefMatrix* modelTransform=0)
- Attach a transform matrix which is applied after the camera look at.
The attached matrix can work in two ways, either as transform of the eye
into the model coordinates - EYE_TO_MODEL, or as a transform of the
model to the eye - MODEL_TO_EYE. The former is equivalent to attaching
a camera internal to the scene graph. The later is equivalent to adding
a osg::Transform at root of the scene to move the scene to the eye point.
Typical used in conjunction with the LookAt position set to home,
in which case it is simply treated as a model view matrix.
If the same behavior as IRIS Performer's setViewMat is desired
then set the LookAt to be (0,0,0),(0,1,0),(0,0,1) since Performer's
default direction is along the y axis, unlike OpenGL and the default OSG.
If modelTransfor is NULL then do not use any model transform - just use the
basic LookAt values.
note: Camera internals maintains the both EYE_TO_MODEL and MODEL_TO_EYE
internally and ensures that they are the inverse of one another.
Matrix* getTransform(TransformMode mode)
const Matrix* getTransform(TransformMode mode) const
enum FusionDistanceMode
PROPORTIONAL_TO_LOOK_DISTANCE
PROPORTIONAL_TO_SCREEN_DISTANCE
void setFusionDistanceMode(FusionDistanceMode mode)
- Set the mode of the fusion distance function which in use to calculate the
fusion distance used in stereo rendering. Default value is
PROPORTIONAL_TO_LOOK_DISTANCE. Use in conjunction with setFusionDistanceRatio(float).
FusionDistanceMode getFusionDistanceMode() const
- Get the mode of the fusion distance function
void setFusionDistanceRatio(float ratio)
- Set the ratio of the fusion distance function which in use to calculate the
fusion distance used in stereo rendering. Default value is 1.0f
Use in conjunction with setFusionDistanceMode(..).
float getFusionDistanceRatio() const
- Get the ratio of the fusion distance function
float getFusionDistance() const
- Calculate and return the fusion distance, using the FusionDistanceFunction
void setScreenDistance(float screenDistance)
- Set the physical distance between the viewers eyes and the display system.
Note, only used when rendering in stereo.
float getScreenDistance() const
- Get the physical distance between the viewers eyes and the display system
Matrix getProjectionMatrix() const
- Get the Projection Matrix
Matrix getModelViewMatrix() const
- Get the ModelView matrix.
If a ModelTransform is supplied then the ModelView matrix is
created by multiplying the current LookAt by ModelTransform.
Otherwise it is simply created by using the current LookAt,
equivalent to using gluLookAt.
inline Polytope getViewFrustum() const
- Get the camera view frustum
virtual ~Camera()
void copy(const Camera&)
ProjectionType _projectionType
AdjustAspectRatioMode _adjustAspectRatioMode
double _left
double _right
double _bottom
double _top
double _zNear
double _zFar
LookAtType _lookAtType
Vec3 _eye
Vec3 _center
Vec3 _up
TransformMode _attachedTransformMode
ref_ptr<RefMatrix> _eyeToModelTransform
ref_ptr<RefMatrix> _modelToEyeTransform
float _screenDistance
FusionDistanceMode _fusionDistanceMode
float _fusionDistanceRatio
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|