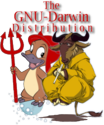
|
Inheritance:
Public Methods-
RefMatrix()
-
RefMatrix( const Matrix& other)
-
RefMatrix( const RefMatrix& other)
-
explicit RefMatrix( float const* const def )
-
RefMatrix( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp&) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
Protected Methods-
virtual ~RefMatrix()
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Inherited from Matrix:
Public Methods-
int compare(const Matrix& m) const
-
bool operator < (const Matrix& m) const
-
bool operator == (const Matrix& m) const
-
bool operator != (const Matrix& m) const
-
inline float& operator()(int row, int col)
-
inline float operator()(int row, int col) const
-
inline bool valid() const
-
inline bool isNaN() const
-
inline void set(const Matrix& other)
-
inline void set(float const* const ptr)
-
void set( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
-
float* ptr() const
-
void makeIdentity()
-
void makeScale( const Vec3& )
-
void makeScale( float, float, float )
-
void makeTranslate( const Vec3& )
-
void makeTranslate( float, float, float )
-
void makeRotate( const Vec3& from, const Vec3& to )
-
void makeRotate( float angle, const Vec3& axis )
-
void makeRotate( float angle, float x, float y, float z )
-
void makeRotate( const Quat& )
-
void makeRotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
void makeOrtho(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline void makeOrtho2D(double left, double right, double bottom, double top)
-
void makeFrustum(double left, double right, double bottom, double top, double zNear, double zFar)
-
void makePerspective(double fovy, double aspectRatio, double zNear, double zFar)
-
void makeLookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
-
bool invert( const Matrix& )
-
inline static Matrix identity( void )
-
inline static Matrix scale( const Vec3& sv)
-
inline static Matrix scale( float sx, float sy, float sz)
-
inline static Matrix translate( const Vec3& dv)
-
inline static Matrix translate( float x, float y, float z)
-
inline static Matrix rotate( const Vec3& from, const Vec3& to)
-
inline static Matrix rotate( float angle, float x, float y, float z)
-
inline static Matrix rotate( float angle, const Vec3& axis)
-
inline static Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
inline static Matrix rotate( const Quat& quat)
-
inline static Matrix inverse( const Matrix& matrix)
-
inline static Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline static Matrix ortho2D(double left, double right, double bottom, double top)
-
inline static Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline static Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
-
inline static Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
-
void setTrans( float tx, float ty, float tz )
-
void setTrans( const Vec3& v )
-
inline Vec3 getTrans() const
-
inline Vec3 getScale() const
-
inline static Vec3 transform3x3(const Vec3& v, const Matrix& m)
-
inline static Vec3 transform3x3(const Matrix& m, const Vec3& v)
-
void mult( const Matrix&, const Matrix& )
-
void preMult( const Matrix& )
-
void postMult( const Matrix& )
-
inline void operator *= ( const Matrix& other )
-
inline Matrix operator * ( const Matrix &m ) const
-
inline Matrix identity(void)
-
inline Matrix scale(float sx, float sy, float sz)
-
inline Matrix scale(const Vec3& v )
-
inline Matrix translate(float tx, float ty, float tz)
-
inline Matrix translate(const Vec3& v )
-
inline Matrix rotate( const Quat& q )
-
inline Matrix rotate(float angle, float x, float y, float z )
-
inline Matrix rotate(float angle, const Vec3& axis )
-
inline Matrix rotate( float angle1, const Vec3& axis1, float angle2, const Vec3& axis2, float angle3, const Vec3& axis3)
-
inline Matrix rotate(const Vec3& from, const Vec3& to )
-
inline Matrix inverse( const Matrix& matrix)
-
inline Matrix ortho(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline Matrix ortho2D(double left, double right, double bottom, double top)
-
inline Matrix frustum(double left, double right, double bottom, double top, double zNear, double zFar)
-
inline Matrix perspective(double fovy, double aspectRatio, double zNear, double zFar)
-
inline Matrix lookAt(const Vec3& eye, const Vec3& center, const Vec3& up)
-
inline Vec3 postMult( const Vec3& v ) const
-
inline Vec3 preMult( const Vec3& v ) const
-
inline Vec4 postMult( const Vec4& v ) const
-
inline Vec4 preMult( const Vec4& v ) const
-
inline Vec3 transform3x3(const Vec3& v, const Matrix& m)
-
inline Vec3 transform3x3(const Matrix& m, const Vec3& v)
-
inline Vec3 operator* (const Vec3& v) const
-
inline Vec4 operator* (const Vec4& v) const
Protected Fields-
float _mat[4][4]
Documentation
RefMatrix()
RefMatrix( const Matrix& other)
RefMatrix( const RefMatrix& other)
explicit RefMatrix( float const* const def )
RefMatrix( float a00, float a01, float a02, float a03, float a10, float a11, float a12, float a13, float a20, float a21, float a22, float a23, float a30, float a31, float a32, float a33)
virtual Object* cloneType() const
virtual Object* clone(const CopyOp&) const
virtual bool isSameKindAs(const Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
virtual ~RefMatrix()
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|