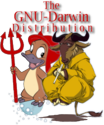
|
Texture base class which encapsulates OpenGl texture functionality which common betweent the various types of OpenGL textures
Inheritance:
Public Methods-
Texture()
-
Texture(const Texture& text, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
virtual osg::Object* cloneType() const = 0
-
virtual osg::Object* clone(const CopyOp& copyop) const = 0
-
virtual bool isSameKindAs(const osg::Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual Type getType() const
-
virtual bool isTextureAttribute() const
-
void setWrap(WrapParameter which, WrapMode wrap)
- Set the texture wrap mode
-
WrapMode getWrap(WrapParameter which) const
- Get the texture wrap mode
-
void setBorderColor(const Vec4& color)
- Sets the border color for this texture.
-
const Vec4& getBorderColor() const
-
void setFilter(FilterParameter which, FilterMode filter)
- Set the texture filter mode
-
FilterMode getFilter(FilterParameter which) const
- Get the texture filter mode
-
void setMaxAnisotropy(float anis)
- Set the maximum anisotropy value, default value is 10 for no anisotropic filtering.
-
inline float getMaxAnisotropy() const
- Get the maximum anisotropy value
-
inline void setInternalFormatMode(InternalFormatMode mode)
- Set the internal format mode.
-
inline InternalFormatMode getInternalFormatMode() const
- Get the internal format mode
-
inline void setInternalFormat(GLint internalFormat)
- Set the internal format to use when creating OpenGL textures.
-
inline GLint getInternalFormat() const
- Get the internal format to use when creating OpenGL textures
-
bool isCompressedInternalFormat() const
-
inline GLuint& getTextureObject(uint contextID) const
- return the OpenGL texture object for specified context
-
inline uint& getModifiedTag(uint contextID) const
-
inline uint& getTextureParameterDirty(uint contextID) const
-
void dirtyTextureObject()
- Force a recompile on next apply() of associated OpenGL texture objects
-
void dirtyTextureParameters()
- Force a resetting on next apply() of associated OpenGL texture parameters
-
static void deleteTextureObject(uint contextID, GLuint handle)
- use deleteTextureObject instead of glDeleteTextures to allow OpenGL texture objects to cached until they can be deleted by the OpenGL context in which they were created, specified by contextID
-
static void flushDeletedTextureObjects(uint contextID)
- flush all the cached display list which need to be deleted in the OpenGL context related to contextID
-
virtual void apply(State& state) const = 0
- Texture is pure virtual base class, apply must be overriden.
-
virtual void compile(State& state) const
- Calls apply(state) to compile the texture.
-
static const Extensions* getExtensions(uint contextID, bool createIfNotInitalized)
- Function to call to get the extension of a specified context.
-
static void setExtensions(uint contextID, Extensions* extensions)
- setExtensions allows users to override the extensions across graphics contexts.
Public Members-
enum WrapParameter
-
enum WrapMode
-
enum FilterParameter
-
enum FilterMode
-
enum InternalFormatMode
-
Get the handle to the texture object for the current context
-
class Extensions: public osg::Referenced
- Extensions class which encapsulates the querring of extensions and associated function pointers, and provide convinience wrappers to check for the extensions or use the associated functions
Protected Fields-
mutable TextureNameList _handleList
-
mutable ImageModifiedTag _modifiedTag
-
mutable TexParameterDirtyList _texParametersDirtyList
-
WrapMode _wrap_s
-
WrapMode _wrap_t
-
WrapMode _wrap_r
-
FilterMode _min_filter
-
FilterMode _mag_filter
-
float _maxAnisotropy
-
Vec4 _borderColor
-
InternalFormatMode _internalFormatMode
-
mutable GLint _internalFormat
Protected Methods-
virtual ~Texture()
-
virtual void computeInternalFormat() const = 0
-
void computeInternalFormatWithImage(osg::Image& image) const
-
bool isCompressedInternalFormat(GLint internalFormat) const
-
void applyTexParameters(GLenum target, State& state) const
- Helper method which does setting of texture paramters.
-
void applyTexImage2D(GLenum target, Image* image, State& state, GLsizei& width, GLsizei& height, GLsizei& numMimpmapLevels) const
- Helper method which does the creation of the texture itself, and does not set or use texture binding.
-
int compareTexture(const Texture& rhs) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
Protected Members-
typedef buffered_value<GLuint> TextureNameList
-
typedef buffered_value<uint> ImageModifiedTag
-
typedef buffered_value<uint> TexParameterDirtyList
Public Methods-
virtual int compare(const StateAttribute& sa) const
-
bool operator < (const StateAttribute& rhs) const
-
bool operator == (const StateAttribute& rhs) const
-
bool operator != (const StateAttribute& rhs) const
-
virtual void getAssociatedModes(std::vector<GLMode>& ) const
Public Members-
typedef GLenum GLMode
-
typedef unsigned int GLModeValue
-
typedef unsigned int OverrideValue
-
enum Values
-
typedef unsigned int Type
-
enum Types
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Texture base class which encapsulates OpenGl texture functionality which common betweent the various types of OpenGL textures
Texture()
Texture(const Texture& text, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
virtual osg::Object* cloneType() const = 0
virtual osg::Object* clone(const CopyOp& copyop) const = 0
virtual bool isSameKindAs(const osg::Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
virtual Type getType() const
virtual bool isTextureAttribute() const
enum WrapParameter
WRAP_S
WRAP_T
WRAP_R
enum WrapMode
CLAMP
CLAMP_TO_EDGE
CLAMP_TO_BORDER
REPEAT
MIRROR
void setWrap(WrapParameter which, WrapMode wrap)
- Set the texture wrap mode
WrapMode getWrap(WrapParameter which) const
- Get the texture wrap mode
void setBorderColor(const Vec4& color)
- Sets the border color for this texture. Makes difference only if
wrap mode is CLAMP_TO_BORDER
const Vec4& getBorderColor() const
enum FilterParameter
MIN_FILTER
MAG_FILTER
enum FilterMode
LINEAR
LINEAR_MIPMAP_LINEAR
LINEAR_MIPMAP_NEAREST
NEAREST
NEAREST_MIPMAP_LINEAR
NEAREST_MIPMAP_NEAREST
void setFilter(FilterParameter which, FilterMode filter)
- Set the texture filter mode
FilterMode getFilter(FilterParameter which) const
- Get the texture filter mode
void setMaxAnisotropy(float anis)
- Set the maximum anisotropy value, default value is 10 for
no anisotropic filtering. If hardware does not support anisotropic
filtering then normal filtering is used, equivilant to a max anisotropy value of 1.0.
valid range is 1.0f upwards. The maximum value depends on the graphics
system being used.
inline float getMaxAnisotropy() const
- Get the maximum anisotropy value
enum InternalFormatMode
USE_IMAGE_DATA_FORMAT
USE_USER_DEFINED_FORMAT
USE_ARB_COMPRESSION
USE_S3TC_DXT1_COMPRESSION
USE_S3TC_DXT3_COMPRESSION
USE_S3TC_DXT5_COMPRESSION
inline void setInternalFormatMode(InternalFormatMode mode)
- Set the internal format mode.
Note, If the mode is set USE_IMAGE_DATA_FORMAT, USE_ARB_COMPRESSION,
USE_S3TC_COMPRESSION the internalFormat is automatically selected,
and will overwrite the previous _internalFormat.
inline InternalFormatMode getInternalFormatMode() const
- Get the internal format mode
inline void setInternalFormat(GLint internalFormat)
- Set the internal format to use when creating OpenGL textures.
Also sets the internalFormatMode to USE_USER_DEFINED_FORMAT.
inline GLint getInternalFormat() const
- Get the internal format to use when creating OpenGL textures
bool isCompressedInternalFormat() const
Get the handle to the texture object for the current context
- Get the handle to the texture object for the current context
inline GLuint& getTextureObject(uint contextID) const
- return the OpenGL texture object for specified context
inline uint& getModifiedTag(uint contextID) const
inline uint& getTextureParameterDirty(uint contextID) const
void dirtyTextureObject()
- Force a recompile on next apply() of associated OpenGL texture objects
void dirtyTextureParameters()
- Force a resetting on next apply() of associated OpenGL texture parameters
static void deleteTextureObject(uint contextID, GLuint handle)
- use deleteTextureObject instead of glDeleteTextures to allow
OpenGL texture objects to cached until they can be deleted
by the OpenGL context in which they were created, specified
by contextID
static void flushDeletedTextureObjects(uint contextID)
- flush all the cached display list which need to be deleted
in the OpenGL context related to contextID
virtual void apply(State& state) const = 0
- Texture is pure virtual base class, apply must be overriden.
virtual void compile(State& state) const
- Calls apply(state) to compile the texture.
static const Extensions* getExtensions(uint contextID, bool createIfNotInitalized)
- Function to call to get the extension of a specified context.
If the Exentsion object for that context has not yet been created then
and the 'createIfNotInitalized' flag been set to false then returns NULL.
If 'createIfNotInitalized' is true then the Extensions object is
automatically created. However, in this case the extension object
only be created with the graphics context associated with ContextID..
static void setExtensions(uint contextID, Extensions* extensions)
- setExtensions allows users to override the extensions across graphics contexts.
typically used when you have different extensions supported across graphics pipes
but need to ensure that they all use the same low common denominator extensions.
virtual ~Texture()
virtual void computeInternalFormat() const = 0
void computeInternalFormatWithImage(osg::Image& image) const
bool isCompressedInternalFormat(GLint internalFormat) const
void applyTexParameters(GLenum target, State& state) const
- Helper method which does setting of texture paramters.
void applyTexImage2D(GLenum target, Image* image, State& state, GLsizei& width, GLsizei& height, GLsizei& numMimpmapLevels) const
- Helper method which does the creation of the texture itself, and
does not set or use texture binding.
int compareTexture(const Texture& rhs) const
- return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs
typedef buffered_value<GLuint> TextureNameList
mutable TextureNameList _handleList
typedef buffered_value<uint> ImageModifiedTag
mutable ImageModifiedTag _modifiedTag
typedef buffered_value<uint> TexParameterDirtyList
mutable TexParameterDirtyList _texParametersDirtyList
WrapMode _wrap_s
WrapMode _wrap_t
WrapMode _wrap_r
FilterMode _min_filter
FilterMode _mag_filter
float _maxAnisotropy
Vec4 _borderColor
InternalFormatMode _internalFormatMode
mutable GLint _internalFormat
- Direct child classes:
- TextureCubeMap
Texture3D
Texture2D
Texture1D
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|