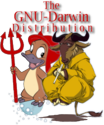
|
A CullStack class which accumulates the current project, modelview matrices and the CullingSet.
Inheritance:
Public Methods-
CullStack()
-
~CullStack()
-
void reset()
-
void setOccluderList(const ShadowVolumeOccluderList& svol)
-
ShadowVolumeOccluderList& getOccluderList()
-
const ShadowVolumeOccluderList& getOccluderList() const
-
void pushViewport(osg::Viewport* viewport)
-
void popViewport()
-
void pushProjectionMatrix(osg::RefMatrix* matrix)
-
void popProjectionMatrix()
-
void pushModelViewMatrix(osg::RefMatrix* matrix)
-
void popModelViewMatrix()
-
inline float getFrustumVolume()
-
void setCullingMode(CullingMode mode)
- Sets the current CullingMode
-
CullingMode getCullingMode() const
- Returns the current CullingMode
-
void setLODScale(float bias)
-
float getLODScale() const
-
void setSmallFeatureCullingPixelSize(float value)
-
float getSmallFeatureCullingPixelSize() const
-
float pixelSize(const Vec3& v, float radius) const
- Compute the pixel of an object at position v, with specified radius
-
float pixelSize(const BoundingSphere& bs) const
- Compute the pixel of an bounding sphere
-
inline void disableAndPushOccludersCurrentMask(NodePath& nodePath)
-
inline void popOccludersCurrentMask(NodePath& nodePath)
-
inline bool isCulled(const std::vector<Vec3>& vertices)
-
inline bool isCulled(const BoundingBox& bb)
-
inline bool isCulled(const BoundingSphere& bs)
-
inline bool isCulled(const osg::Node& node)
-
inline void pushCurrentMask()
-
inline void popCurrentMask()
-
CullingStack& getClipSpaceCullingStack()
-
CullingStack& getProjectionCullingStack()
-
CullingStack& getModelViewCullingStack()
-
CullingSet& getCurrentCullingSet()
-
inline osg::Viewport* getViewport()
-
inline osg::RefMatrix& getModelViewMatrix()
-
inline osg::RefMatrix& getProjectionMatrix()
-
inline osg::Matrix getWindowMatrix()
-
inline const osg::RefMatrix& getMVPW()
-
inline const osg::Vec3& getEyeLocal() const
-
inline const osg::Vec3 getUpLocal() const
-
inline const osg::Vec3 getLookVectorLocal() const
-
inline Viewport* getViewport()
-
inline RefMatrix& getModelViewMatrix()
-
inline RefMatrix& getProjectionMatrix()
-
inline Matrix getWindowMatrix()
-
inline const RefMatrix& getMVPW()
-
inline RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
Public Members-
typedef std::vector<ShadowVolumeOccluder> OccluderList
-
enum CullingModeValues
-
typedef unsigned int CullingMode
-
typedef fast_back_stack<ref_ptr<CullingSet> > CullingStack
Protected Fields-
CullingMode _cullingMode
-
float _LODScale
-
float _smallFeatureCullingPixelSize
-
ShadowVolumeOccluderList _occluderList
-
MatrixStack _projectionStack
-
MatrixStack _modelviewStack
-
MatrixStack _MVPW_Stack
-
ViewportStack _viewportStack
-
EyePointStack _eyePointStack
-
CullingStack _clipspaceCullingStack
-
CullingStack _projectionCullingStack
-
CullingStack _modelviewCullingStack
-
float _frustumVolume
-
unsigned int _bbCornerNear
-
unsigned int _bbCornerFar
-
ref_ptr<osg::RefMatrix> _identity
-
MatrixList _reuseMatrixList
-
unsigned int _currentReuseMatrixIndex
Protected Methods-
void pushCullingSet()
-
void popCullingSet()
-
void computeFrustumVolume()
-
inline osg::RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
Protected Members-
typedef fast_back_stack< ref_ptr<RefMatrix> > MatrixStack
-
typedef fast_back_stack<ref_ptr<Viewport> > ViewportStack
-
typedef fast_back_stack<Vec3> EyePointStack
-
typedef std::vector< osg::ref_ptr<osg::RefMatrix> > MatrixList
Documentation
A CullStack class which accumulates the current project, modelview matrices
and the CullingSet.
CullStack()
~CullStack()
typedef std::vector<ShadowVolumeOccluder> OccluderList
enum CullingModeValues
NO_CULLING
VIEW_FRUSTUM_CULLING
NEAR_PLANE_CULLING
FAR_PLANE_CULLING
SMALL_FEATURE_CULLING
SHADOW_OCCLUSION_CULLING
ENABLE_ALL_CULLING
typedef unsigned int CullingMode
void reset()
void setOccluderList(const ShadowVolumeOccluderList& svol)
ShadowVolumeOccluderList& getOccluderList()
const ShadowVolumeOccluderList& getOccluderList() const
void pushViewport(osg::Viewport* viewport)
void popViewport()
void pushProjectionMatrix(osg::RefMatrix* matrix)
void popProjectionMatrix()
void pushModelViewMatrix(osg::RefMatrix* matrix)
void popModelViewMatrix()
inline float getFrustumVolume()
void setCullingMode(CullingMode mode)
- Sets the current CullingMode
CullingMode getCullingMode() const
- Returns the current CullingMode
void setLODScale(float bias)
float getLODScale() const
void setSmallFeatureCullingPixelSize(float value)
float getSmallFeatureCullingPixelSize() const
float pixelSize(const Vec3& v, float radius) const
- Compute the pixel of an object at position v, with specified radius
float pixelSize(const BoundingSphere& bs) const
- Compute the pixel of an bounding sphere
inline void disableAndPushOccludersCurrentMask(NodePath& nodePath)
inline void popOccludersCurrentMask(NodePath& nodePath)
inline bool isCulled(const std::vector<Vec3>& vertices)
inline bool isCulled(const BoundingBox& bb)
inline bool isCulled(const BoundingSphere& bs)
inline bool isCulled(const osg::Node& node)
inline void pushCurrentMask()
inline void popCurrentMask()
typedef fast_back_stack<ref_ptr<CullingSet> > CullingStack
CullingStack& getClipSpaceCullingStack()
CullingStack& getProjectionCullingStack()
CullingStack& getModelViewCullingStack()
CullingSet& getCurrentCullingSet()
inline osg::Viewport* getViewport()
inline osg::RefMatrix& getModelViewMatrix()
inline osg::RefMatrix& getProjectionMatrix()
inline osg::Matrix getWindowMatrix()
inline const osg::RefMatrix& getMVPW()
inline const osg::Vec3& getEyeLocal() const
inline const osg::Vec3 getUpLocal() const
inline const osg::Vec3 getLookVectorLocal() const
void pushCullingSet()
void popCullingSet()
CullingMode _cullingMode
float _LODScale
float _smallFeatureCullingPixelSize
ShadowVolumeOccluderList _occluderList
typedef fast_back_stack< ref_ptr<RefMatrix> > MatrixStack
MatrixStack _projectionStack
MatrixStack _modelviewStack
MatrixStack _MVPW_Stack
typedef fast_back_stack<ref_ptr<Viewport> > ViewportStack
ViewportStack _viewportStack
typedef fast_back_stack<Vec3> EyePointStack
EyePointStack _eyePointStack
CullingStack _clipspaceCullingStack
CullingStack _projectionCullingStack
CullingStack _modelviewCullingStack
void computeFrustumVolume()
float _frustumVolume
unsigned int _bbCornerNear
unsigned int _bbCornerFar
ref_ptr<osg::RefMatrix> _identity
typedef std::vector< osg::ref_ptr<osg::RefMatrix> > MatrixList
MatrixList _reuseMatrixList
unsigned int _currentReuseMatrixIndex
inline osg::RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
inline Viewport* getViewport()
inline RefMatrix& getModelViewMatrix()
inline RefMatrix& getProjectionMatrix()
inline Matrix getWindowMatrix()
inline const RefMatrix& getMVPW()
inline RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
- Direct child classes:
- CollectOccludersVisitor
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|