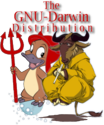
|
Base class for all internal nodes in the scene graph.
Inheritance:
Public Methods-
Node()
- Construct a node.
-
Node(const Node&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
virtual Object* cloneType() const
- clone the an object of the same type as the node
-
virtual Object* clone(const CopyOp& copyop) const
- return a clone of a node, with Object* return type
-
virtual bool isSameKindAs(const Object* obj) const
- return true if this and obj are of the same kind of object
-
virtual const char* libraryName() const
- return the name of the node's library
-
virtual const char* className() const
- return the name of the node's class type
-
virtual Group* asGroup()
- convert 'this' into a Group pointer if Node is a Group, otherwise return 0.
-
virtual const Group* asGroup() const
- convert 'const this' into a const Group pointer if Node is a Group, otherwise return 0.
-
virtual Transform* asTransform()
- convert 'this' into a Transform pointer if Node is a Transform, otherwise return 0.
-
virtual const Transform* asTransform() const
- convert 'const this' into a const Transform pointer if Node is a Transform, otherwise return 0.
-
virtual void accept(NodeVisitor& nv)
- Visitor Pattern : calls the apply method of a NodeVisitor with this node's type
-
virtual void ascend(NodeVisitor& nv)
- Traverse upwards : calls parents' accept method with NodeVisitor
-
virtual void traverse(NodeVisitor& )
- Traverse downwards : calls children's accept method with NodeVisitor
-
inline void setName( const std::string& name )
- Set the name of node using C++ style string
-
inline void setName( const char* name )
- Set the name of node using a C style string
-
inline const std::string& getName() const
- Get the name of node
-
inline const ParentList& getParents() const
- Get the parent list of node.
-
inline ParentList getParents()
- Get the a copy of parent list of node.
-
inline Group* getParent(unsigned int i)
-
inline const Group* getParent(unsigned int i) const
- Get a single const parent of node.
-
inline unsigned int getNumParents() const
- Get the number of parents of node.
-
void setUpdateCallback(NodeCallback* nc)
- Set update node callback, called during update traversal.
-
inline NodeCallback* getUpdateCallback()
- Get update node callback, called during update traversal.
-
inline const NodeCallback* getUpdateCallback() const
- Get const update node callback, called during update traversal.
-
void setAppCallback(NodeCallback* nc)
- deprecated.
-
inline NodeCallback* getAppCallback()
- deprecated.
-
inline const NodeCallback* getAppCallback() const
- deprecated.
-
inline unsigned int getNumChildrenRequiringUpdateTraversal() const
- Get the number of Children of this node which require App traversal, since they have an AppCallback attached to them or their children
-
void setCullCallback(NodeCallback* nc)
- Set cull node callback, called during cull traversal.
-
inline NodeCallback* getCullCallback()
- Get cull node callback, called during cull traversal.
-
inline const NodeCallback* getCullCallback() const
- Get const cull node callback, called during cull traversal.
-
void setCullingActive(bool active)
- Set the view frustum/small feature culling of this node to be active or inactive.
-
inline bool getCullingActive() const
- Get the view frustum/small feature _cullingActive flag for this node.
-
inline unsigned int getNumChildrenWithCullingDisabled() const
- Get the number of Children of this node which have culling disabled
-
inline bool isCullingActive() const
- Return true if this node can be culled by view frustum, occlusion or small feature culling during the cull traversal.
-
inline unsigned int getNumChildrenWithOccluderNodes() const
- Get the number of Children of this node which are or have OccluderNode's
-
bool containsOccluderNodes() const
- return true if this node is an OccluderNode or the subgraph below this node are OccluderNodes
-
inline void setNodeMask(NodeMask nm)
- Set the node mask.
-
inline NodeMask getNodeMask() const
- Get the node Mask.
-
inline const DescriptionList& getDescriptions() const
- Get the description list of the const node
-
inline DescriptionList& getDescriptions()
- Get the description list of the const node
-
inline const std::string& getDescription(unsigned int i) const
- Get a single const description of the const node
-
inline std::string& getDescription(unsigned int i)
- Get a single description of the node
-
inline unsigned int getNumDescriptions() const
- Get the number of descriptions of the node
-
void addDescription(const std::string& desc)
- Add a description string to the node
-
inline void setStateSet(osg::StateSet* dstate)
- set the node's StateSet
-
osg::StateSet* getOrCreateStateSet()
- return the node's StateSet, if one does not already exist create it set the node and return the newly created StateSet.
-
inline osg::StateSet* getStateSet()
- return the node's StateSet.
-
inline const osg::StateSet* getStateSet() const
- return the node's const StateSet.
-
inline const BoundingSphere& getBound() const
- get the bounding sphere of node.
-
void dirtyBound()
- Mark this node's bounding sphere dirty.
Public Members-
typedef std::vector<Group*> ParentList
- A vector of osg::Group pointers which is used to store the parent(s) of node
-
typedef unsigned int NodeMask
-
typedef std::vector<std::string> DescriptionList
- A vector of std::string's which are used to describe the object
Protected Fields-
mutable BoundingSphere _bsphere
-
mutable bool _bsphere_computed
-
std::string _name
-
ParentList _parents
-
ref_ptr<NodeCallback> _updateCallback
-
unsigned int _numChildrenRequiringUpdateTraversal
-
ref_ptr<NodeCallback> _cullCallback
-
bool _cullingActive
-
unsigned int _numChildrenWithCullingDisabled
-
unsigned int _numChildrenWithOccluderNodes
-
NodeMask _nodeMask
-
DescriptionList _descriptions
-
ref_ptr<StateSet> _stateset
Protected Methods-
virtual ~Node()
- Node destructor.
-
virtual bool computeBound() const
- Compute the bounding sphere around Node's geometry or children.
-
void addParent(osg::Group* node)
-
void removeParent(osg::Group* node)
-
void setNumChildrenRequiringUpdateTraversal(unsigned int num)
-
void setNumChildrenWithCullingDisabled(unsigned int num)
-
void setNumChildrenWithOccluderNodes(unsigned int num)
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Base class for all internal nodes in the scene graph.
Provides interface for most common node operations (Composite Pattern).
Node()
- Construct a node.
Initialize the parent list to empty, node name to "" and
bounding sphere dirty flag to true.
Node(const Node&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
virtual Object* cloneType() const
- clone the an object of the same type as the node
virtual Object* clone(const CopyOp& copyop) const
- return a clone of a node, with Object* return type
virtual bool isSameKindAs(const Object* obj) const
- return true if this and obj are of the same kind of object
virtual const char* libraryName() const
- return the name of the node's library
virtual const char* className() const
- return the name of the node's class type
virtual Group* asGroup()
- convert 'this' into a Group pointer if Node is a Group, otherwise return 0.
Equivalent to dynamic_cast(this).
virtual const Group* asGroup() const
- convert 'const this' into a const Group pointer if Node is a Group, otherwise return 0.
Equivalent to dynamic_cast(this).
virtual Transform* asTransform()
- convert 'this' into a Transform pointer if Node is a Transform, otherwise return 0.
Equivalent to dynamic_cast(this).
virtual const Transform* asTransform() const
- convert 'const this' into a const Transform pointer if Node is a Transform, otherwise return 0.
Equivalent to dynamic_cast(this).
virtual void accept(NodeVisitor& nv)
- Visitor Pattern : calls the apply method of a NodeVisitor with this node's type
virtual void ascend(NodeVisitor& nv)
- Traverse upwards : calls parents' accept method with NodeVisitor
virtual void traverse(NodeVisitor& )
- Traverse downwards : calls children's accept method with NodeVisitor
inline void setName( const std::string& name )
- Set the name of node using C++ style string
inline void setName( const char* name )
- Set the name of node using a C style string
inline const std::string& getName() const
- Get the name of node
typedef std::vector<Group*> ParentList
- A vector of osg::Group pointers which is used to store the parent(s) of node
inline const ParentList& getParents() const
- Get the parent list of node.
inline ParentList getParents()
- Get the a copy of parent list of node. A copy is returned to
prevent modification of the parent list.
inline Group* getParent(unsigned int i)
inline const Group* getParent(unsigned int i) const
-
Get a single const parent of node.
- Parameters:
- i - index of the parent to get.
- Returns:
- the parent i.
inline unsigned int getNumParents() const
-
Get the number of parents of node.
- Returns:
- the number of parents of this node.
void setUpdateCallback(NodeCallback* nc)
- Set update node callback, called during update traversal.
inline NodeCallback* getUpdateCallback()
- Get update node callback, called during update traversal.
inline const NodeCallback* getUpdateCallback() const
- Get const update node callback, called during update traversal.
void setAppCallback(NodeCallback* nc)
- deprecated.
inline NodeCallback* getAppCallback()
- deprecated.
inline const NodeCallback* getAppCallback() const
- deprecated.
inline unsigned int getNumChildrenRequiringUpdateTraversal() const
- Get the number of Children of this node which require App traversal,
since they have an AppCallback attached to them or their children
void setCullCallback(NodeCallback* nc)
- Set cull node callback, called during cull traversal.
inline NodeCallback* getCullCallback()
- Get cull node callback, called during cull traversal.
inline const NodeCallback* getCullCallback() const
- Get const cull node callback, called during cull traversal.
void setCullingActive(bool active)
- Set the view frustum/small feature culling of this node to be active or inactive.
The default value to true for _cullingActive. Used a guide
to the cull traversal.
inline bool getCullingActive() const
- Get the view frustum/small feature _cullingActive flag for this node. Used a guide
to the cull traversal.
inline unsigned int getNumChildrenWithCullingDisabled() const
- Get the number of Children of this node which have culling disabled
inline bool isCullingActive() const
- Return true if this node can be culled by view frustum, occlusion or small feature culling during the cull traversal.
note, return true only if no children have culling disabled, and the local _cullingActive flag is true.
inline unsigned int getNumChildrenWithOccluderNodes() const
- Get the number of Children of this node which are or have OccluderNode's
bool containsOccluderNodes() const
- return true if this node is an OccluderNode or the subgraph below this node are OccluderNodes
typedef unsigned int NodeMask
inline void setNodeMask(NodeMask nm)
- Set the node mask. Note, node mask is will be replaced by TraversalMask.
inline NodeMask getNodeMask() const
- Get the node Mask. Note, node mask is will be replaced by TraversalMask.
typedef std::vector<std::string> DescriptionList
- A vector of std::string's which are used to describe the object
inline const DescriptionList& getDescriptions() const
- Get the description list of the const node
inline DescriptionList& getDescriptions()
- Get the description list of the const node
inline const std::string& getDescription(unsigned int i) const
- Get a single const description of the const node
inline std::string& getDescription(unsigned int i)
- Get a single description of the node
inline unsigned int getNumDescriptions() const
- Get the number of descriptions of the node
void addDescription(const std::string& desc)
- Add a description string to the node
inline void setStateSet(osg::StateSet* dstate)
- set the node's StateSet
osg::StateSet* getOrCreateStateSet()
- return the node's StateSet, if one does not already exist create it
set the node and return the newly created StateSet. This ensures
that a valid StateSet is always returned and can be used directly.
inline osg::StateSet* getStateSet()
- return the node's StateSet. returns NULL if a stateset is not attached.
inline const osg::StateSet* getStateSet() const
- return the node's const StateSet. returns NULL if a stateset is not attached.
inline const BoundingSphere& getBound() const
- get the bounding sphere of node.
Using lazy evaluation computes the bounding sphere if it is 'dirty'.
void dirtyBound()
- Mark this node's bounding sphere dirty.
Forcing it to be computed on the next call to getBound().
virtual ~Node()
- Node destructor. Note, is protected so that Nodes cannot
be deleted other than by being dereferenced and the reference
count being zero (see osg::Referenced), preventing the deletion
of nodes which are still in use. This also means that
Node's cannot be created on stack i.e Node node will not compile,
forcing all nodes to be created on the heap i.e Node* node
= new Node().
virtual bool computeBound() const
- Compute the bounding sphere around Node's geometry or children.
This method is automatically called by getBound() when the bounding
sphere has been marked dirty via dirtyBound().
mutable BoundingSphere _bsphere
mutable bool _bsphere_computed
std::string _name
void addParent(osg::Group* node)
void removeParent(osg::Group* node)
ParentList _parents
ref_ptr<NodeCallback> _updateCallback
unsigned int _numChildrenRequiringUpdateTraversal
void setNumChildrenRequiringUpdateTraversal(unsigned int num)
ref_ptr<NodeCallback> _cullCallback
bool _cullingActive
unsigned int _numChildrenWithCullingDisabled
void setNumChildrenWithCullingDisabled(unsigned int num)
unsigned int _numChildrenWithOccluderNodes
void setNumChildrenWithOccluderNodes(unsigned int num)
NodeMask _nodeMask
DescriptionList _descriptions
ref_ptr<StateSet> _stateset
- Direct child classes:
- Group
Geode
- Friends:
- class osg::Group
class osg::Drawable
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|