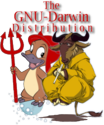
|
Inheritance:
Public Methods-
CollectOccludersVisitor()
-
virtual ~CollectOccludersVisitor()
-
virtual CollectOccludersVisitor* cloneType() const
-
virtual void reset()
-
virtual float getDistanceToEyePoint(const Vec3& pos, bool withLODScale) const
-
virtual float getDistanceFromEyePoint(const Vec3& pos, bool withLODScale) const
-
virtual void apply(osg::Node&)
-
virtual void apply(osg::Transform& node)
-
virtual void apply(osg::Projection& node)
-
virtual void apply(osg::Switch& node)
-
virtual void apply(osg::LOD& node)
-
virtual void apply(osg::OccluderNode& node)
-
void setMinimumShadowOccluderVolume(float vol)
-
float getMinimumShadowOccluderVolume() const
-
void setCreateDrawablesOnOccludeNodes(bool flag)
-
bool getCreateDrawablesOnOccludeNodes() const
-
void setCollectedOcculderList(const ShadowVolumeOccluderSet& svol)
-
ShadowVolumeOccluderSet& getCollectedOccluderSet()
-
const ShadowVolumeOccluderSet& getCollectedOccluderSet() const
-
void removeOccludedOccluders()
- remove occluded occluders for the collected occluders list
Public Members-
typedef std::set<ShadowVolumeOccluder> ShadowVolumeOccluderSet
Protected Fields-
float _minimumShadowOccluderVolume
-
bool _createDrawables
-
ShadowVolumeOccluderSet _occluderSet
Protected Methods-
CollectOccludersVisitor(const CollectOccludersVisitor&)
- prevent unwanted copy construction
-
CollectOccludersVisitor& operator = (const CollectOccludersVisitor&)
- prevent unwanted copy operator
-
inline void handle_cull_callbacks_and_traverse(osg::Node& node)
-
inline void handle_cull_callbacks_and_accept(osg::Node& node, osg::Node* acceptNode)
Public Methods-
inline void setVisitorType(VisitorType type)
-
inline VisitorType getVisitorType() const
-
inline void setTraversalNumber(int fn)
-
inline int getTraversalNumber() const
-
inline void setFrameStamp(FrameStamp* fs)
-
inline const FrameStamp* getFrameStamp() const
-
inline void setTraversalMask(Node::NodeMask mask)
-
inline Node::NodeMask getTraversalMask() const
-
inline void setNodeMaskOverride(Node::NodeMask mask)
-
inline Node::NodeMask getNodeMaskOverride() const
-
inline bool validNodeMask(const osg::Node& node) const
-
inline void setTraversalMode(TraversalMode mode)
-
inline TraversalMode getTraversalMode() const
-
inline void traverse(Node& node)
-
inline void pushOntoNodePath(Node* node)
-
inline void popFromNodePath()
-
NodePath& getNodePath()
-
const NodePath& getNodePath() const
-
virtual bool getLocalToWorldMatrix(Matrix& matrix, Node* node)
-
virtual bool getWorldToLocalMatrix(Matrix& matrix, Node* node)
-
virtual osg::Vec3 getEyePoint() const
Public Members-
enum TraversalMode
-
enum VisitorType
Protected Fields-
VisitorType _visitorType
-
int _traversalNumber
-
ref_ptr<FrameStamp> _frameStamp
-
TraversalMode _traversalMode
-
Node::NodeMask _traversalMask
-
Node::NodeMask _nodeMaskOverride
-
NodePath _nodePath
Public Methods-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Public Methods-
void setOccluderList(const ShadowVolumeOccluderList& svol)
-
ShadowVolumeOccluderList& getOccluderList()
-
const ShadowVolumeOccluderList& getOccluderList() const
-
void pushViewport(osg::Viewport* viewport)
-
void popViewport()
-
void pushProjectionMatrix(osg::RefMatrix* matrix)
-
void popProjectionMatrix()
-
void pushModelViewMatrix(osg::RefMatrix* matrix)
-
void popModelViewMatrix()
-
inline float getFrustumVolume()
-
void setCullingMode(CullingMode mode)
-
CullingMode getCullingMode() const
-
void setLODScale(float bias)
-
float getLODScale() const
-
void setSmallFeatureCullingPixelSize(float value)
-
float getSmallFeatureCullingPixelSize() const
-
float pixelSize(const Vec3& v, float radius) const
-
float pixelSize(const BoundingSphere& bs) const
-
inline void disableAndPushOccludersCurrentMask(NodePath& nodePath)
-
inline void popOccludersCurrentMask(NodePath& nodePath)
-
inline bool isCulled(const std::vector<Vec3>& vertices)
-
inline bool isCulled(const BoundingBox& bb)
-
inline bool isCulled(const BoundingSphere& bs)
-
inline bool isCulled(const osg::Node& node)
-
inline void pushCurrentMask()
-
inline void popCurrentMask()
-
CullingStack& getClipSpaceCullingStack()
-
CullingStack& getProjectionCullingStack()
-
CullingStack& getModelViewCullingStack()
-
CullingSet& getCurrentCullingSet()
-
inline osg::Viewport* getViewport()
-
inline osg::RefMatrix& getModelViewMatrix()
-
inline osg::RefMatrix& getProjectionMatrix()
-
inline osg::Matrix getWindowMatrix()
-
inline const osg::RefMatrix& getMVPW()
-
inline const osg::Vec3& getEyeLocal() const
-
inline const osg::Vec3 getUpLocal() const
-
inline const osg::Vec3 getLookVectorLocal() const
-
inline Viewport* getViewport()
-
inline RefMatrix& getModelViewMatrix()
-
inline RefMatrix& getProjectionMatrix()
-
inline Matrix getWindowMatrix()
-
inline const RefMatrix& getMVPW()
-
inline RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
Public Members-
typedef std::vector<ShadowVolumeOccluder> OccluderList
-
enum CullingModeValues
-
typedef unsigned int CullingMode
-
typedef fast_back_stack<ref_ptr<CullingSet> > CullingStack
Protected Fields-
CullingMode _cullingMode
-
float _LODScale
-
float _smallFeatureCullingPixelSize
-
ShadowVolumeOccluderList _occluderList
-
MatrixStack _projectionStack
-
MatrixStack _modelviewStack
-
MatrixStack _MVPW_Stack
-
ViewportStack _viewportStack
-
EyePointStack _eyePointStack
-
CullingStack _clipspaceCullingStack
-
CullingStack _projectionCullingStack
-
CullingStack _modelviewCullingStack
-
float _frustumVolume
-
unsigned int _bbCornerNear
-
unsigned int _bbCornerFar
-
ref_ptr<osg::RefMatrix> _identity
-
MatrixList _reuseMatrixList
-
unsigned int _currentReuseMatrixIndex
Protected Methods-
void pushCullingSet()
-
void popCullingSet()
-
void computeFrustumVolume()
-
inline osg::RefMatrix* createOrReuseMatrix(const osg::Matrix& value)
Protected Members-
typedef fast_back_stack< ref_ptr<RefMatrix> > MatrixStack
-
typedef fast_back_stack<ref_ptr<Viewport> > ViewportStack
-
typedef fast_back_stack<Vec3> EyePointStack
-
typedef std::vector< osg::ref_ptr<osg::RefMatrix> > MatrixList
Documentation
typedef std::set<ShadowVolumeOccluder> ShadowVolumeOccluderSet
CollectOccludersVisitor()
virtual ~CollectOccludersVisitor()
virtual CollectOccludersVisitor* cloneType() const
virtual void reset()
virtual float getDistanceToEyePoint(const Vec3& pos, bool withLODScale) const
virtual float getDistanceFromEyePoint(const Vec3& pos, bool withLODScale) const
virtual void apply(osg::Node&)
virtual void apply(osg::Transform& node)
virtual void apply(osg::Projection& node)
virtual void apply(osg::Switch& node)
virtual void apply(osg::LOD& node)
virtual void apply(osg::OccluderNode& node)
void setMinimumShadowOccluderVolume(float vol)
float getMinimumShadowOccluderVolume() const
void setCreateDrawablesOnOccludeNodes(bool flag)
bool getCreateDrawablesOnOccludeNodes() const
void setCollectedOcculderList(const ShadowVolumeOccluderSet& svol)
ShadowVolumeOccluderSet& getCollectedOccluderSet()
const ShadowVolumeOccluderSet& getCollectedOccluderSet() const
void removeOccludedOccluders()
- remove occluded occluders for the collected occluders list
CollectOccludersVisitor(const CollectOccludersVisitor&)
- prevent unwanted copy construction
CollectOccludersVisitor& operator = (const CollectOccludersVisitor&)
- prevent unwanted copy operator
inline void handle_cull_callbacks_and_traverse(osg::Node& node)
inline void handle_cull_callbacks_and_accept(osg::Node& node, osg::Node* acceptNode)
float _minimumShadowOccluderVolume
bool _createDrawables
ShadowVolumeOccluderSet _occluderSet
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|