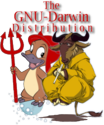
|
Pure virtual base class for drawable Geometry.
Inheritance:
Public Methods-
Drawable()
-
Drawable(const Drawable& drawable, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual Geometry* asGeometry()
- convert 'this' into a Geometry pointer if Drawable is a Geometry, otherwise return 0.
-
virtual const Geometry* asGeometry() const
- convert 'const this' into a const Geometry pointer if Drawable is a Geometry, otherwise return 0.
-
inline const ParentList& getParents() const
- Get the parent list of drawable.
-
inline ParentList getParents()
- Get the a copy of parent list of node.
-
inline Node* getParent(unsigned int i)
- Get a single parent of Drawable.
-
inline const Node* getParent(unsigned int i) const
- Get a single const parent of Drawable.
-
inline unsigned int getNumParents() const
- Get the number of parents of node.
-
inline void setStateSet(StateSet* state)
- Set the StateSet attached to the Drawable.
-
inline StateSet* getStateSet()
- Get the attached StateSet
-
inline const StateSet* getStateSet() const
- Get the attached const StateSet
-
StateSet* getOrCreateStateSet()
- Get the attached const StateSet, if one is not already attach create one, attach it to the drawable and return a pointer to it
-
void dirtyBound()
- Dirty the bounding box, forcing a computeBound() on the next call to getBound().
-
inline const BoundingBox& getBound() const
- get bounding box of geoset.
-
inline void setShape(Shape* shape)
- Set the Shape of the drawable.
-
inline Shape* getShape()
- Get the Shape of the Drawable
-
inline const Shape* getShape() const
- Get the const Shape of the const Drawable
-
void setSupportsDisplayList(bool flag)
- Set the drawable to it can or cannot be used in conjunction with OpenGL display lists.
-
inline bool getSupportsDisplayList() const
- Get whether display lists are supported for this drawable instance
-
void setUseDisplayList(bool flag)
- When set to true, force the draw method to use OpenGL Display List for rendering.
-
inline bool getUseDisplayList() const
- Return whether OpenGL display lists are being used for rendering
-
void dirtyDisplayList()
- Force a recompile on next draw() of any OpenGL display list associated with this geoset
-
virtual void compile(State& state) const
- Immediately compile this drawable into an OpenGL Display List.
-
void setUpdateCallback(UpdateCallback* ac)
- Set the UpdateCallback which allows users to attach customize the undating of an object during the app traversal
-
UpdateCallback* getUpdateCallback()
- Get the non const UpdateCallback
-
void setAppCallback(AppCallback* ac)
- deprecated
-
AppCallback* getAppCallback()
- deprecated
-
const AppCallback* getAppCallback() const
- deprecated
-
void setCullCallback(CullCallback* cc)
- Set the CullCallback which allows users to attach customize the culling of Drawable during the cull traversal
-
CullCallback* getCullCallback()
- Get the non const CullCallback
-
const CullCallback* getCullCallback() const
- Get the const CullCallback
-
void setDrawCallback(DrawCallback* dc)
- Set the DrawCallback which allows users to attach customize the drawing of existing Drawable object
-
DrawCallback* getDrawCallback()
- Get the non const DrawCallback
-
const DrawCallback* getDrawCallback() const
- Get the const DrawCallback
-
virtual void drawImplementation(State& state) const = 0
- draw directly ignoring an OpenGL display list which could be attached.
-
static void deleteDisplayList(uint contextID, uint globj)
- use deleteDisplayList instead of glDeleteList to allow OpenGL display list to cached until they can be deleted by the OpenGL context in which they were created, specified by contextID
-
static void flushDeletedDisplayLists(uint contextID)
- flush all the cached display list which need to be deleted in the OpenGL context related to contextID
-
virtual bool supports(AttributeFunctor&) const
- return true if the Drawable subclass supports accept(AttributeFunctor&)
-
virtual void accept(AttributeFunctor&)
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
-
virtual bool supports(ConstAttributeFunctor&) const
- return true if the Drawable subclass supports accept(ConstAttributeFunctor&)
-
virtual void accept(ConstAttributeFunctor&) const
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
-
virtual bool supports(PrimitiveFunctor&) const
- return true if the Drawable subclass supports accept(PrimitiveFunctor&)
-
virtual void accept(PrimitiveFunctor&) const
- accept a PrimtiveFunctor and call its methods to tell it about the interal primtives that this Drawable has.
-
inline void draw(State& state) const
- draw OpenGL primitives.
Public Members-
typedef std::vector<Node*> ParentList
- A vector of osg::Node pointers which is used to store the parent(s) of drawable
-
struct UpdateCallback: public virtual osg::Referenced
-
struct AppCallback: public UpdateCallback
-
struct CullCallback: public virtual osg::Referenced
-
struct DrawCallback: public virtual osg::Referenced
- Callback attached to an Drawable which allows the users to customize the drawing of an exist Drawable object.
-
enum AttributeType
-
class AttributeFunctor
-
class ConstAttributeFunctor
-
class PrimitiveFunctor
Protected Fields-
ParentList _parents
-
ref_ptr<StateSet> _stateset
-
mutable BoundingBox _bbox
-
mutable bool _bbox_computed
-
ref_ptr<Shape> _shape
-
bool _supportsDisplayList
-
bool _useDisplayList
-
mutable GLObjectList _globjList
-
ref_ptr<UpdateCallback> _updateCallback
-
ref_ptr<DrawCallback> _drawCallback
-
ref_ptr<CullCallback> _cullCallback
Protected Methods-
Drawable& operator = (const Drawable&)
-
virtual ~Drawable()
-
virtual bool computeBound() const
- compute the bounding box of the drawable.
-
void addParent(osg::Node* node)
-
void removeParent(osg::Node* node)
Protected Members-
typedef osg::buffered_value<uint> GLObjectList
Inherited from Object:
Public Methods-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp&) const
-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Pure virtual base class for drawable Geometry. Contains no drawing primitives
directly, these are provided by subclasses such as osg::Geometry. State attributes
for a Drawable are maintained in StateSet which the Drawable maintains
a referenced counted pointer to. Both Drawable's and StateSet's can
be shared for optimal memory usage and graphics performance.
Drawable()
Drawable(const Drawable& drawable, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
virtual bool isSameKindAs(const Object* obj) const
virtual const char* libraryName() const
virtual const char* className() const
virtual Geometry* asGeometry()
- convert 'this' into a Geometry pointer if Drawable is a Geometry, otherwise return 0.
Equivalent to dynamic_cast(this).
virtual const Geometry* asGeometry() const
- convert 'const this' into a const Geometry pointer if Drawable is a Geometry, otherwise return 0.
Equivalent to dynamic_cast(this).
typedef std::vector<Node*> ParentList
- A vector of osg::Node pointers which is used to store the parent(s) of drawable
inline const ParentList& getParents() const
- Get the parent list of drawable.
inline ParentList getParents()
- Get the a copy of parent list of node. A copy is returned to
prevent modification of the parent list.
inline Node* getParent(unsigned int i)
- Get a single parent of Drawable.
- Parameters:
- i - index of the parent to get.
- Returns:
- the parent i.
inline const Node* getParent(unsigned int i) const
- Get a single const parent of Drawable.
- Parameters:
- i - index of the parent to get.
- Returns:
- the parent i.
inline unsigned int getNumParents() const
-
Get the number of parents of node.
- Returns:
- the number of parents of this node.
inline void setStateSet(StateSet* state)
- Set the StateSet attached to the Drawable.
Previously attached StateSet are automatically unreferenced on
assignment of a new drawstate.
inline StateSet* getStateSet()
- Get the attached StateSet
inline const StateSet* getStateSet() const
- Get the attached const StateSet
StateSet* getOrCreateStateSet()
- Get the attached const StateSet,
if one is not already attach create one,
attach it to the drawable and return a pointer to it
void dirtyBound()
- Dirty the bounding box, forcing a computeBound() on the next call
to getBound(). Should be called in the internal geometry of the Drawable
is modified.
inline const BoundingBox& getBound() const
- get bounding box of geoset.
Note, now made virtual to make it possible to implement user-drawn
objects albeit so what crudely, to be improved later.
inline void setShape(Shape* shape)
- Set the Shape of the drawable. The shape can be used to
speed up collision detection or as a guide for produral
geometry generation - see osg::ProduralGeometry.
inline Shape* getShape()
- Get the Shape of the Drawable
inline const Shape* getShape() const
- Get the const Shape of the const Drawable
void setSupportsDisplayList(bool flag)
- Set the drawable to it can or cannot be used in conjunction with OpenGL
display lists. With set to true, calls to Drawable::setUseDisplayList,
whereas when set to false, no display lists can be created and calls
to setUseDisplayList are ignored, and a warning is produced. The later
is typically used to guard against the switching on of display lists
on objects with dynamic internal data such as continuous Level of Detail
algorithms.
inline bool getSupportsDisplayList() const
- Get whether display lists are supported for this drawable instance
void setUseDisplayList(bool flag)
- When set to true, force the draw method to use OpenGL Display List for rendering.
If false rendering directly. If the display list has not been already
compile the next call to draw will automatically create the display list.
inline bool getUseDisplayList() const
- Return whether OpenGL display lists are being used for rendering
void dirtyDisplayList()
- Force a recompile on next draw() of any OpenGL display list associated with this geoset
virtual void compile(State& state) const
- Immediately compile this drawable into an OpenGL Display List.
Note I, operation is ignored if _useDisplayList to false.
Note II, compile is not intended to be overridden in subclasses.
struct UpdateCallback: public virtual osg::Referenced
virtual void update(osg::NodeVisitor* visitor, osg::Drawable* drawable) = 0
- do customized app code
void setUpdateCallback(UpdateCallback* ac)
- Set the UpdateCallback which allows users to attach customize the undating of an object during the app traversal
UpdateCallback* getUpdateCallback()
- Get the non const UpdateCallback
struct AppCallback: public UpdateCallback
virtual void app(osg::NodeVisitor* visitor, osg::Drawable* drawable) = 0
- do customized app code
virtual void update(osg::NodeVisitor* visitor, osg::Drawable* drawable)
void setAppCallback(AppCallback* ac)
- deprecated
AppCallback* getAppCallback()
- deprecated
const AppCallback* getAppCallback() const
- deprecated
struct CullCallback: public virtual osg::Referenced
virtual bool cull(osg::NodeVisitor* visitor, osg::Drawable* drawable, osg::State* state=NULL) const = 0
- do customized cull code
void setCullCallback(CullCallback* cc)
- Set the CullCallback which allows users to attach customize the culling of Drawable during the cull traversal
CullCallback* getCullCallback()
- Get the non const CullCallback
const CullCallback* getCullCallback() const
- Get the const CullCallback
struct DrawCallback: public virtual osg::Referenced
- Callback attached to an Drawable which allows the users to customize the drawing of an exist Drawable object.
The draw callback is implement as a replacement to the Drawable's own drawImplementation() method, if the
the user intends to decorate the exist draw code then simple call the drawable->drawImplementation() from
with the callbacks drawImplementation() method. This allows the users to do both pre and post callbacks
without fuss and can even diable the inner draw in required.
virtual void drawImplementation(State& state, const osg::Drawable* drawable) const = 0
- do customized draw code
void setDrawCallback(DrawCallback* dc)
- Set the DrawCallback which allows users to attach customize the drawing of existing Drawable object
DrawCallback* getDrawCallback()
- Get the non const DrawCallback
const DrawCallback* getDrawCallback() const
- Get the const DrawCallback
virtual void drawImplementation(State& state) const = 0
- draw directly ignoring an OpenGL display list which could be attached.
This is the internal draw method which does the drawing itself,
and is the method to override when deriving from Drawable.
static void deleteDisplayList(uint contextID, uint globj)
- use deleteDisplayList instead of glDeleteList to allow
OpenGL display list to cached until they can be deleted
by the OpenGL context in which they were created, specified
by contextID
static void flushDeletedDisplayLists(uint contextID)
- flush all the cached display list which need to be deleted
in the OpenGL context related to contextID
enum AttributeType
VERTICES
NORMALS
COLORS
TEXTURE_COORDS
TEXTURE_COORDS_0
TEXTURE_COORDS_1
TEXTURE_COORDS_2
TEXTURE_COORDS_3
TEXTURE_COORDS_4
TEXTURE_COORDS_5
TEXTURE_COORDS_6
TEXTURE_COORDS_7
virtual bool supports(AttributeFunctor&) const
- return true if the Drawable subclass supports accept(AttributeFunctor&)
virtual void accept(AttributeFunctor&)
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
return true if functor handled by drawable, return false on failure of drawable to generate functor calls.
virtual bool supports(ConstAttributeFunctor&) const
- return true if the Drawable subclass supports accept(ConstAttributeFunctor&)
virtual void accept(ConstAttributeFunctor&) const
- accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
return true if functor handled by drawable, return false on failure of drawable to generate functor calls.
virtual bool supports(PrimitiveFunctor&) const
- return true if the Drawable subclass supports accept(PrimitiveFunctor&)
virtual void accept(PrimitiveFunctor&) const
- accept a PrimtiveFunctor and call its methods to tell it about the interal primtives that this Drawable has.
return true if functor handled by drawable, return false on failure of drawable to generate functor calls.
Note, PrimtiveFunctor only provide const access of the primtives, as primitives may be procedurally generated
so one cannot modify it.
Drawable& operator = (const Drawable&)
virtual ~Drawable()
virtual bool computeBound() const
- compute the bounding box of the drawable. Method must be
implemented by subclasses.
void addParent(osg::Node* node)
void removeParent(osg::Node* node)
ParentList _parents
ref_ptr<StateSet> _stateset
mutable BoundingBox _bbox
mutable bool _bbox_computed
ref_ptr<Shape> _shape
bool _supportsDisplayList
bool _useDisplayList
typedef osg::buffered_value<uint> GLObjectList
mutable GLObjectList _globjList
ref_ptr<UpdateCallback> _updateCallback
ref_ptr<DrawCallback> _drawCallback
ref_ptr<CullCallback> _cullCallback
inline void draw(State& state) const
- draw OpenGL primitives.
If the drawable has _useDisplayList set to true then use an OpenGL display
list, automatically compiling one if required.
Otherwise call drawImplementation().
Note, draw method should *not* be overridden in subclasses as it
manages the optional display list.
- Direct child classes:
- ShapeDrawable
ImpostorSprite
Geometry
GeoSet
DrawPixels
- Friends:
- class Node
class Geode
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|