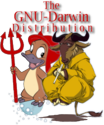
|
Leaf Node for defining the position of ClipPlanes in the scene
Inheritance:
Public Methods-
ClipNode()
-
ClipNode(const ClipNode& es, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
-
META_Node(osg, ClipNode)
-
void createClipBox(const BoundingBox& bb, unsigned int clipPlaneNumberBase=0)
- Create a 6 clip planes to create a clip box
-
bool addClipPlane(ClipPlane* clipplane)
- Add a ClipPlane to a ClipNode.
-
bool removeClipPlane(ClipPlane* clipplane)
- Remove ClipPlane from a ClipNode.
-
bool removeClipPlane(unsigned int pos)
- Remove ClipPlane, at specified index, from a ClipNode.
-
inline unsigned int getNumClipPlanes() const
- return the number of ClipPlanes
-
inline ClipPlane* getClipPlane(unsigned int pos)
- Get ClipPlane at specificed index position
-
inline const ClipPlane* getClipPlane(unsigned int pos) const
- Get const ClipPlane at specificed index position
-
inline ClipPlaneList& getClipPlaneList()
- Get the ClipPlaneList
-
inline const ClipPlaneList& getClipPlaneList() const
- Get the const ClipPlaneList
-
void setStateSetModes(StateSet&, StateAttribute::GLModeValue) const
- Set the GLModes on StateSet associated with the ClipPlanes
-
void setLocalStateSetModes(StateAttribute::GLModeValue=StateAttribute::ON)
- Set up the local StateSet
Public Members-
typedef std::vector<ref_ptr<ClipPlane> > ClipPlaneList
Protected Fields-
StateAttribute::GLModeValue _value
-
ClipPlaneList _planes
Protected Methods-
virtual ~ClipNode()
-
virtual bool computeBound() const
Inherited from Group:
Public Methods-
virtual Group* asGroup()
-
virtual const Group* asGroup() const
-
virtual void traverse(NodeVisitor& nv)
-
virtual bool addChild( Node* child )
-
virtual bool removeChild( Node* child )
-
virtual bool removeChild(unsigned int pos, unsigned int numChildrenToRemove=1)
-
virtual bool replaceChild( Node* origChild, Node* newChild )
-
inline unsigned int getNumChildren() const
-
virtual bool setChild( unsigned int i, Node* node )
-
inline Node* getChild( unsigned int i )
-
inline const Node* getChild( unsigned int i ) const
-
inline bool containsNode( const Node* node ) const
-
inline unsigned int getChildIndex( const Node* node ) const
Public Members-
typedef std::vector<ref_ptr<Node> > ChildList
Protected Fields-
ChildList _children
Inherited from Node:
Public Methods-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp& copyop) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual Transform* asTransform()
-
virtual const Transform* asTransform() const
-
virtual void accept(NodeVisitor& nv)
-
virtual void ascend(NodeVisitor& nv)
-
inline void setName( const std::string& name )
-
inline void setName( const char* name )
-
inline const std::string& getName() const
-
inline const ParentList& getParents() const
-
inline ParentList getParents()
-
inline Group* getParent(unsigned int i)
-
inline const Group* getParent(unsigned int i) const
-
inline unsigned int getNumParents() const
-
void setUpdateCallback(NodeCallback* nc)
-
inline NodeCallback* getUpdateCallback()
-
inline const NodeCallback* getUpdateCallback() const
-
void setAppCallback(NodeCallback* nc)
-
inline NodeCallback* getAppCallback()
-
inline const NodeCallback* getAppCallback() const
-
inline unsigned int getNumChildrenRequiringUpdateTraversal() const
-
void setCullCallback(NodeCallback* nc)
-
inline NodeCallback* getCullCallback()
-
inline const NodeCallback* getCullCallback() const
-
void setCullingActive(bool active)
-
inline bool getCullingActive() const
-
inline unsigned int getNumChildrenWithCullingDisabled() const
-
inline bool isCullingActive() const
-
inline unsigned int getNumChildrenWithOccluderNodes() const
-
bool containsOccluderNodes() const
-
inline void setNodeMask(NodeMask nm)
-
inline NodeMask getNodeMask() const
-
inline const DescriptionList& getDescriptions() const
-
inline DescriptionList& getDescriptions()
-
inline const std::string& getDescription(unsigned int i) const
-
inline std::string& getDescription(unsigned int i)
-
inline unsigned int getNumDescriptions() const
-
void addDescription(const std::string& desc)
-
inline void setStateSet(osg::StateSet* dstate)
-
osg::StateSet* getOrCreateStateSet()
-
inline osg::StateSet* getStateSet()
-
inline const osg::StateSet* getStateSet() const
-
inline const BoundingSphere& getBound() const
-
void dirtyBound()
Public Members-
typedef std::vector<Group*> ParentList
-
typedef unsigned int NodeMask
-
typedef std::vector<std::string> DescriptionList
Protected Fields-
mutable BoundingSphere _bsphere
-
mutable bool _bsphere_computed
-
std::string _name
-
ParentList _parents
-
ref_ptr<NodeCallback> _updateCallback
-
unsigned int _numChildrenRequiringUpdateTraversal
-
ref_ptr<NodeCallback> _cullCallback
-
bool _cullingActive
-
unsigned int _numChildrenWithCullingDisabled
-
unsigned int _numChildrenWithOccluderNodes
-
NodeMask _nodeMask
-
DescriptionList _descriptions
-
ref_ptr<StateSet> _stateset
Protected Methods-
void addParent(osg::Group* node)
-
void removeParent(osg::Group* node)
-
void setNumChildrenRequiringUpdateTraversal(unsigned int num)
-
void setNumChildrenWithCullingDisabled(unsigned int num)
-
void setNumChildrenWithOccluderNodes(unsigned int num)
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Leaf Node for defining the position of ClipPlanes in the scene
typedef std::vector<ref_ptr<ClipPlane> > ClipPlaneList
ClipNode()
ClipNode(const ClipNode& es, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
META_Node(osg, ClipNode)
void createClipBox(const BoundingBox& bb, unsigned int clipPlaneNumberBase=0)
- Create a 6 clip planes to create a clip box
bool addClipPlane(ClipPlane* clipplane)
- Add a ClipPlane to a ClipNode. Return true if plane is added,
return false if plane already exists in ClipNode, or clipplane is false.
bool removeClipPlane(ClipPlane* clipplane)
- Remove ClipPlane from a ClipNode. Return true if plane is removed,
return false if plane does not exists in ClipNode.
bool removeClipPlane(unsigned int pos)
- Remove ClipPlane, at specified index, from a ClipNode. Return true if plane is removed,
return false if plane does not exists in ClipNode.
inline unsigned int getNumClipPlanes() const
- return the number of ClipPlanes
inline ClipPlane* getClipPlane(unsigned int pos)
- Get ClipPlane at specificed index position
inline const ClipPlane* getClipPlane(unsigned int pos) const
- Get const ClipPlane at specificed index position
inline ClipPlaneList& getClipPlaneList()
- Get the ClipPlaneList
inline const ClipPlaneList& getClipPlaneList() const
- Get the const ClipPlaneList
void setStateSetModes(StateSet&, StateAttribute::GLModeValue) const
- Set the GLModes on StateSet associated with the ClipPlanes
void setLocalStateSetModes(StateAttribute::GLModeValue=StateAttribute::ON)
- Set up the local StateSet
virtual ~ClipNode()
virtual bool computeBound() const
StateAttribute::GLModeValue _value
ClipPlaneList _planes
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|