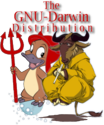
|
Visitor for type safe operations on osg::Node's.
Inheritance:
Public Methods-
NodeVisitor(TraversalMode tm=TRAVERSE_NONE)
-
NodeVisitor(VisitorType type, TraversalMode tm=TRAVERSE_NONE)
-
virtual ~NodeVisitor()
-
virtual void reset()
- Method to call to reset visitor.
-
inline void setVisitorType(VisitorType type)
- Set the VisitorType, used to distingush different visitors during traversal of the scene, typically used in the Node::traverse() method to select which behaviour to use for different types of traversal/visitors
-
inline VisitorType getVisitorType() const
- Get the VisitorType
-
inline void setTraversalNumber(int fn)
- Set the traversal number.
-
inline int getTraversalNumber() const
- Get the traversal number.
-
inline void setFrameStamp(FrameStamp* fs)
- Set the FrameStamp that this traversal is assoicated with
-
inline const FrameStamp* getFrameStamp() const
- Get the FrameStamp that this traversal is assoicated with
-
inline void setTraversalMask(Node::NodeMask mask)
- Set the TraversalMask of this NodeVisitor.
-
inline Node::NodeMask getTraversalMask() const
- Get the TraversalMask
-
inline void setNodeMaskOverride(Node::NodeMask mask)
- Set the NodeMaskOverride mask.
-
inline Node::NodeMask getNodeMaskOverride() const
- Get the NodeMaskOverride mask
-
inline bool validNodeMask(const osg::Node& node) const
- Method to called by Node and its subclass' Node::accept() method, if the result is true to be used to cull operations of nodes and their subgraphs.
-
inline void setTraversalMode(TraversalMode mode)
- Set the traversal mode for Node::traverse() to use when deciding which children of a node to traverse.
-
inline TraversalMode getTraversalMode() const
- Get the traversal mode
-
inline void traverse(Node& node)
- Method for handling traversal of a nodes.
-
inline void pushOntoNodePath(Node* node)
- Method called by osg::Node::accept() method before a call the NodeVisitor::apply().
-
inline void popFromNodePath()
- Method callby osg::Node::accept() method after a call the NodeVisitor::apply().
-
NodePath& getNodePath()
- Get the non const NodePath from the top most node applied down to the current Node being visited
-
const NodePath& getNodePath() const
- Get the const NodePath from the top most node applied down to the current Node being visited
-
virtual bool getLocalToWorldMatrix(Matrix& matrix, Node* node)
- Get the Local To World Matrix from the NodePath for specified Transform::Mode, and u
-
virtual bool getWorldToLocalMatrix(Matrix& matrix, Node* node)
- Get the World To Local Matrix from the NodePath for specified Transform::Mode
-
virtual osg::Vec3 getEyePoint() const
- Get the eye point in local coordinates.
-
virtual float getDistanceToEyePoint(const Vec3& , bool ) const
- Get the distance from a point to the eye point, distance value in local coordinate system.
-
virtual float getDistanceFromEyePoint(const Vec3& , bool ) const
- Get the distance of a point from the eye point, distance value in the eye coordinate system.
-
virtual void apply(Node& node)
-
virtual void apply(Geode& node)
-
virtual void apply(Billboard& node)
-
virtual void apply(Group& node)
-
virtual void apply(Projection& node)
-
virtual void apply(ClipNode& node)
-
virtual void apply(LightSource& node)
-
virtual void apply(Transform& node)
-
virtual void apply(DOFTransform& node)
-
virtual void apply(MatrixTransform& node)
-
virtual void apply(PositionAttitudeTransform& node)
-
virtual void apply(Switch& node)
-
virtual void apply(Sequence& node)
-
virtual void apply(LOD& node)
-
virtual void apply(Impostor& node)
-
virtual void apply(ClearNode& node)
-
virtual void apply(OccluderNode& node)
Public Members-
enum TraversalMode
-
enum VisitorType
Protected Fields-
VisitorType _visitorType
-
int _traversalNumber
-
ref_ptr<FrameStamp> _frameStamp
-
TraversalMode _traversalMode
-
Node::NodeMask _traversalMask
-
Node::NodeMask _nodeMaskOverride
-
NodePath _nodePath
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Visitor for type safe operations on osg::Node's.
Based on GOF's Visitor pattern. The NodeVisitor
is useful for developing type safe operations to nodes
in the scene graph (as per Visitor pattern), and adds to this
support for optional scene graph traversal to allow
operations to be applied to whole scenes at once. The Visitor
pattern uses a technique of double dispatch as a mechanism to
called the appropriate apply(..) method of the NodeVisitor. To
use this feature one must use the Node::accept(NodeVisitor) which
is extended in each Node subclass, rather than the NodeVisitor
apply directly. So use root->accept(myVisitor); instead of
myVisitor.apply(*root). The later method will bypass the double
dispatch and the appropriate NodeVisitor::apply(..) method will
not be called.
enum TraversalMode
TRAVERSE_NONE
TRAVERSE_PARENTS
TRAVERSE_ALL_CHILDREN
TRAVERSE_ACTIVE_CHILDREN
enum VisitorType
NODE_VISITOR
UPDATE_VISITOR
COLLECT_OCCLUDER_VISITOR
CULL_VISITOR
NodeVisitor(TraversalMode tm=TRAVERSE_NONE)
NodeVisitor(VisitorType type, TraversalMode tm=TRAVERSE_NONE)
virtual ~NodeVisitor()
virtual void reset()
- Method to call to reset visitor. Useful for your visitor accumulates
state during a traversal, and you plan to reuse the visitor.
To flush that state for the next traversal than call reset() prior
to each traversal.
inline void setVisitorType(VisitorType type)
- Set the VisitorType, used to distingush different visitors during
traversal of the scene, typically used in the Node::traverse() method
to select which behaviour to use for different types of traversal/visitors
inline VisitorType getVisitorType() const
- Get the VisitorType
inline void setTraversalNumber(int fn)
- Set the traversal number. Typically used to denote the frame count.
inline int getTraversalNumber() const
- Get the traversal number. Typically used to denote the frame count.
inline void setFrameStamp(FrameStamp* fs)
- Set the FrameStamp that this traversal is assoicated with
inline const FrameStamp* getFrameStamp() const
- Get the FrameStamp that this traversal is assoicated with
inline void setTraversalMask(Node::NodeMask mask)
- Set the TraversalMask of this NodeVisitor.
The TraversalMask is used by the NodeVisitor::validNodeMask() method
to determine whether to operate on a node and its subgraph.
validNodeMask() is called automaticaly in the Node::accept() method before
any call to NodeVisitor::apply(), apply() is only ever called if validNodeMask
returns true. Note, if NodeVisitor::_traversalMask is 0 then all operations
will be swithced off for all nodes. Whereas setting both _traversalMask and
_nodeMaskOverride to 0xffffffff will allow a visitor to work on all nodes
regardless of their own Node::_nodeMask state.
inline Node::NodeMask getTraversalMask() const
- Get the TraversalMask
inline void setNodeMaskOverride(Node::NodeMask mask)
- Set the NodeMaskOverride mask.
Used in validNodeMask() to determine whether to operate on a node or its
subgraph, by OR'ing NodeVisitor::_nodeMaskOverride with the Node's own Node::_nodeMask.
Typically used to force on nodes which may have
been switched off by their own Node::_nodeMask.
inline Node::NodeMask getNodeMaskOverride() const
- Get the NodeMaskOverride mask
inline bool validNodeMask(const osg::Node& node) const
- Method to called by Node and its subclass' Node::accept() method, if the result is true
to be used to cull operations of nodes and their subgraphs.
Return true if the result of a bit wise and of the NodeVisitor::_traversalMask
with the bit or between NodeVistor::_nodeMaskOverride and the Node::_nodeMask.
default values for _traversalMask is 0xffffffff, _nodeMaskOverride is 0x0,
and osg::Node::_nodeMask is 0xffffffff.
inline void setTraversalMode(TraversalMode mode)
- Set the traversal mode for Node::traverse() to use when
deciding which children of a node to traverse. If a
NodeVisitor has been attached via setTraverseVisitor()
and the new mode is not TRAVERSE_VISITOR then the attached
visitor is detached. Default mode is TRAVERSE_NONE.
inline TraversalMode getTraversalMode() const
- Get the traversal mode
inline void traverse(Node& node)
- Method for handling traversal of a nodes.
If you intend to use the visitor for actively traversing
the scene graph then make sure the accept() methods call
this method unless they handle traversal directly.
inline void pushOntoNodePath(Node* node)
- Method called by osg::Node::accept() method before
a call the NodeVisitor::apply(). The back of the list will,
therefore, be the current node being visited inside the apply(..),
and the rest of the list will be the parental sequence of nodes
from the top most node applied down the graph to the current node.
Note, the user does not typically call pushNodeOnPath() as it
will be called automatically by the Node::accept() method.
inline void popFromNodePath()
- Method callby osg::Node::accept() method after
a call the NodeVisitor::apply().
Note, the user does not typically call pushNodeOnPath() as it
will be called automatically by the Node::accept() method.
NodePath& getNodePath()
- Get the non const NodePath from the top most node applied down
to the current Node being visited
const NodePath& getNodePath() const
- Get the const NodePath from the top most node applied down
to the current Node being visited
virtual bool getLocalToWorldMatrix(Matrix& matrix, Node* node)
- Get the Local To World Matrix from the NodePath for specified Transform::Mode, and u
virtual bool getWorldToLocalMatrix(Matrix& matrix, Node* node)
- Get the World To Local Matrix from the NodePath for specified Transform::Mode
virtual osg::Vec3 getEyePoint() const
- Get the eye point in local coordinates.
Note, not all NodeVisitor implement this method, it is mainly cull visitors which will implement.
virtual float getDistanceToEyePoint(const Vec3& , bool ) const
- Get the distance from a point to the eye point, distance value in local coordinate system.
Note, not all NodeVisitor implement this method, it is mainly cull visitors which will implement.
If the getDistianceFromEyePoint(pos) is not implmented than a default value of 0.0 is returned.
virtual float getDistanceFromEyePoint(const Vec3& , bool ) const
- Get the distance of a point from the eye point, distance value in the eye coordinate system.
Note, not all NodeVisitor implement this method, it is mainly cull visitors which will implement.
If the getDistianceFromEyePoint(pos) is not implmented than a default value of 0.0 is returned.
virtual void apply(Node& node)
virtual void apply(Geode& node)
virtual void apply(Billboard& node)
virtual void apply(Group& node)
virtual void apply(Projection& node)
virtual void apply(ClipNode& node)
virtual void apply(LightSource& node)
virtual void apply(Transform& node)
virtual void apply(DOFTransform& node)
virtual void apply(MatrixTransform& node)
virtual void apply(PositionAttitudeTransform& node)
virtual void apply(Switch& node)
virtual void apply(Sequence& node)
virtual void apply(LOD& node)
virtual void apply(Impostor& node)
virtual void apply(ClearNode& node)
virtual void apply(OccluderNode& node)
VisitorType _visitorType
int _traversalNumber
ref_ptr<FrameStamp> _frameStamp
TraversalMode _traversalMode
Node::NodeMask _traversalMask
Node::NodeMask _nodeMaskOverride
NodePath _nodePath
- Direct child classes:
- CollectOccludersVisitor
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|