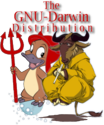
|
A Transform is a group node for which all children are transformed by a 4x4 matrix.
Inheritance:
Public Methods-
Transform()
-
Transform(const Transform&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
META_Node(osg, Transform)
-
virtual Transform* asTransform()
-
virtual const Transform* asTransform() const
-
virtual MatrixTransform* asMatrixTransform()
-
virtual const MatrixTransform* asMatrixTransform() const
-
virtual PositionAttitudeTransform* asPositionAttitudeTransform()
-
virtual const PositionAttitudeTransform* asPositionAttitudeTransform() const
-
virtual DOFTransform* asDOFTransform()
-
virtual const DOFTransform* asDOFTransform() const
-
void setReferenceFrame(ReferenceFrame rf)
- Set the transform's ReferenceFrame, either to be relative to its parent reference frame, or relative to an absolute coordinate frame.
-
ReferenceFrame getReferenceFrame() const
-
void setComputeTransformCallback(ComputeTransformCallback* ctc)
- Set the ComputerTransfromCallback which allows users to attach custom computation of the local transformation as seen by cull traversers and the like.
-
ComputeTransformCallback* getComputeTransformCallback()
- Get the non const ComputerTransfromCallback
-
const ComputeTransformCallback* getComputeTransformCallback() const
- Get the const ComputerTransfromCallback
-
inline bool getLocalToWorldMatrix(Matrix& matrix, NodeVisitor* nv) const
- Get the transformation matrix which moves from local coords to world coords.
-
inline bool getWorldToLocalMatrix(Matrix& matrix, NodeVisitor* nv) const
- Get the transformation matrix which moves from world coords to local coords.
-
virtual bool computeLocalToWorldMatrix(Matrix& matrix, NodeVisitor*) const
-
virtual bool computeWorldToLocalMatrix(Matrix& matrix, NodeVisitor*) const
Public Members-
enum ReferenceFrame
-
struct ComputeTransformCallback: public virtual osg::Referenced
- Callback attached to an Transform to specify how to compute the modelview transformation for the transform below the Transform node.
Protected Fields-
ref_ptr<ComputeTransformCallback> _computeTransformCallback
-
ReferenceFrame _referenceFrame
Protected Methods-
virtual ~Transform()
-
virtual bool computeBound() const
- Overrides Group's computeBound.
Inherited from Group:
Public Methods-
virtual Group* asGroup()
-
virtual const Group* asGroup() const
-
virtual void traverse(NodeVisitor& nv)
-
virtual bool addChild( Node* child )
-
virtual bool removeChild( Node* child )
-
virtual bool removeChild(unsigned int pos, unsigned int numChildrenToRemove=1)
-
virtual bool replaceChild( Node* origChild, Node* newChild )
-
inline unsigned int getNumChildren() const
-
virtual bool setChild( unsigned int i, Node* node )
-
inline Node* getChild( unsigned int i )
-
inline const Node* getChild( unsigned int i ) const
-
inline bool containsNode( const Node* node ) const
-
inline unsigned int getChildIndex( const Node* node ) const
Public Members-
typedef std::vector<ref_ptr<Node> > ChildList
Protected Fields-
ChildList _children
Inherited from Node:
Public Methods-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp& copyop) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual void accept(NodeVisitor& nv)
-
virtual void ascend(NodeVisitor& nv)
-
inline void setName( const std::string& name )
-
inline void setName( const char* name )
-
inline const std::string& getName() const
-
inline const ParentList& getParents() const
-
inline ParentList getParents()
-
inline Group* getParent(unsigned int i)
-
inline const Group* getParent(unsigned int i) const
-
inline unsigned int getNumParents() const
-
void setUpdateCallback(NodeCallback* nc)
-
inline NodeCallback* getUpdateCallback()
-
inline const NodeCallback* getUpdateCallback() const
-
void setAppCallback(NodeCallback* nc)
-
inline NodeCallback* getAppCallback()
-
inline const NodeCallback* getAppCallback() const
-
inline unsigned int getNumChildrenRequiringUpdateTraversal() const
-
void setCullCallback(NodeCallback* nc)
-
inline NodeCallback* getCullCallback()
-
inline const NodeCallback* getCullCallback() const
-
void setCullingActive(bool active)
-
inline bool getCullingActive() const
-
inline unsigned int getNumChildrenWithCullingDisabled() const
-
inline bool isCullingActive() const
-
inline unsigned int getNumChildrenWithOccluderNodes() const
-
bool containsOccluderNodes() const
-
inline void setNodeMask(NodeMask nm)
-
inline NodeMask getNodeMask() const
-
inline const DescriptionList& getDescriptions() const
-
inline DescriptionList& getDescriptions()
-
inline const std::string& getDescription(unsigned int i) const
-
inline std::string& getDescription(unsigned int i)
-
inline unsigned int getNumDescriptions() const
-
void addDescription(const std::string& desc)
-
inline void setStateSet(osg::StateSet* dstate)
-
osg::StateSet* getOrCreateStateSet()
-
inline osg::StateSet* getStateSet()
-
inline const osg::StateSet* getStateSet() const
-
inline const BoundingSphere& getBound() const
-
void dirtyBound()
Public Members-
typedef std::vector<Group*> ParentList
-
typedef unsigned int NodeMask
-
typedef std::vector<std::string> DescriptionList
Protected Fields-
mutable BoundingSphere _bsphere
-
mutable bool _bsphere_computed
-
std::string _name
-
ParentList _parents
-
ref_ptr<NodeCallback> _updateCallback
-
unsigned int _numChildrenRequiringUpdateTraversal
-
ref_ptr<NodeCallback> _cullCallback
-
bool _cullingActive
-
unsigned int _numChildrenWithCullingDisabled
-
unsigned int _numChildrenWithOccluderNodes
-
NodeMask _nodeMask
-
DescriptionList _descriptions
-
ref_ptr<StateSet> _stateset
Protected Methods-
void addParent(osg::Group* node)
-
void removeParent(osg::Group* node)
-
void setNumChildrenRequiringUpdateTraversal(unsigned int num)
-
void setNumChildrenWithCullingDisabled(unsigned int num)
-
void setNumChildrenWithOccluderNodes(unsigned int num)
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
A Transform is a group node for which all children are transformed by
a 4x4 matrix. It is often used for positioning objects within a scene,
producing trackball functionality or for animation.
Transform itself does not provide set/get functions, only the interface
for defining what the 4x4 transformation is. Subclasses, such as
MatrixTransform and PositionAttitudeTransform support the use of an
osg::Matrix or a osg::Vec3/osg::Quat resprectively.
The Transform node can be customized via the ComputeTransfromCallback
which can be attached to the node. This might be used to convert from
internal representations of the transformation into generic osg::Matrix
objects which are used during scene grpah traversal, such as
CullTraversal and IntersectionTraversal.
Note: if the transformation matrix scales the subgraph then the normals
of the underlying geometry will need to be renormalized to be unit
vectors once more. This can be done transparently through OpenGL's
use of either GL_NORMALIZE and GL_SCALE_NORMALIZE modes. For further
background reading see the glNormalize documentation in the OpenGL
Reference Guide (the blue book). To enable it in the OSG, you simply
need to attach a local osg::StateSet to the osg::Transform, and set
the appropriate mode to ON via
stateset->setMode(GL_NORMALIZE, osg::StateAttribute::ON);
Transform()
Transform(const Transform&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
META_Node(osg, Transform)
virtual Transform* asTransform()
virtual const Transform* asTransform() const
virtual MatrixTransform* asMatrixTransform()
virtual const MatrixTransform* asMatrixTransform() const
virtual PositionAttitudeTransform* asPositionAttitudeTransform()
virtual const PositionAttitudeTransform* asPositionAttitudeTransform() const
virtual DOFTransform* asDOFTransform()
virtual const DOFTransform* asDOFTransform() const
enum ReferenceFrame
RELATIVE_TO_PARENTS
RELATIVE_TO_ABSOLUTE
void setReferenceFrame(ReferenceFrame rf)
- Set the transform's ReferenceFrame, either to be relative to its
parent reference frame, or relative to an absolute coordinate
frame. RELATIVE_TO_PARENTS is the default.
Note: setting the ReferenceFrame to be RELATIVE_TO_ABSOLUTE will
also set the CullingActive flag on the transform, and hence all
of its parents, to false, thereby disabling culling of it and
all its parents. This is neccessary to prevent inappropriate
culling, but may impact cull times if the absolute transform is
deep in the scene graph. It is therefore recommend to only use
absolute Transforms at the top of the scene, for such things as
heads up displays.
ReferenceFrame getReferenceFrame() const
struct ComputeTransformCallback: public virtual osg::Referenced
- Callback attached to an Transform to specify how to compute the
modelview transformation for the transform below the Transform
node.
virtual bool computeLocalToWorldMatrix(Matrix& matrix, const Transform* transform, NodeVisitor* nv) const = 0
- Get the transformation matrix which moves from local coords
to world coords
virtual bool computeWorldToLocalMatrix(Matrix& matrix, const Transform* transform, NodeVisitor* nv) const = 0
- Get the transformation matrix which moves from world coords
to local coords
void setComputeTransformCallback(ComputeTransformCallback* ctc)
- Set the ComputerTransfromCallback which allows users to attach
custom computation of the local transformation as seen by cull
traversers and the like.
ComputeTransformCallback* getComputeTransformCallback()
- Get the non const ComputerTransfromCallback
const ComputeTransformCallback* getComputeTransformCallback() const
- Get the const ComputerTransfromCallback
inline bool getLocalToWorldMatrix(Matrix& matrix, NodeVisitor* nv) const
- Get the transformation matrix which moves from local coords to
world coords.
Returns true if the Matrix passed in has been updated.
inline bool getWorldToLocalMatrix(Matrix& matrix, NodeVisitor* nv) const
- Get the transformation matrix which moves from world coords to
local coords.
Return true if the Matrix passed in has been updated.
virtual bool computeLocalToWorldMatrix(Matrix& matrix, NodeVisitor*) const
virtual bool computeWorldToLocalMatrix(Matrix& matrix, NodeVisitor*) const
virtual ~Transform()
virtual bool computeBound() const
- Overrides Group's computeBound.
There is no need to override in subclasses from osg::Transform
since this computeBound() uses the underlying matrix (calling
computeMatrix if required.)
ref_ptr<ComputeTransformCallback> _computeTransformCallback
ReferenceFrame _referenceFrame
- Direct child classes:
- PositionAttitudeTransform
MatrixTransform
DOFTransform
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|